Java: Check if two binary trees are identical or not
Java Basic: Exercise-182 with Solution
Write a Java program to check if two binary trees are identical. Assume that two binary trees have the same structure and every identical position has the same value.
Sample Solution:
Java Code:
// Importing necessary Java utilities
import java.util.*;
// Main class Solution
public class Solution {
// Main method
public static void main(String[] args) {
// Creating TreeNode 'a'
TreeNode a = new TreeNode(1);
a.left = new TreeNode(2);
a.right = new TreeNode(3);
a.left.left = new TreeNode(4);
// Creating TreeNode 'b'
TreeNode b = new TreeNode(1);
b.left = new TreeNode(2);
b.right = new TreeNode(3);
b.left.right = new TreeNode(4);
// Creating TreeNode 'c'
TreeNode c = new TreeNode(1);
c.left = new TreeNode(2);
c.right = new TreeNode(3);
c.left.right = new TreeNode(4);
// Comparing TreeNode 'a' and TreeNode 'b'
System.out.println("\nComparing TreeNode a and TreeNode b:");
System.out.println(is_Identical_tree_node(a, b));
// Comparing TreeNode 'b' and TreeNode 'c'
System.out.println("\nComparing TreeNode b and TreeNode c:");
System.out.println(is_Identical_tree_node(b, c));
}
// Method to check if two TreeNode objects are identical
public static boolean is_Identical_tree_node(TreeNode a, TreeNode b) {
// Write your code here
if (a == null && b == null) return true;
if (a == null || b == null) {
return false;
}
if (a.val != b.val) return false;
return is_Identical_tree_node(a.left, b.left) &&
is_Identical_tree_node(a.right, b.right);
}
}
// Definition of TreeNode class
class TreeNode {
public int val;
public TreeNode left, right;
// Constructor to initialize TreeNode object with a value
public TreeNode(int val) {
this.val = val;
this.left = this.right = null;
}
}
Sample Output:
Comparing TreeNode a and TreeNode b: false Comparing TreeNode b and TreeNode c: true
Flowchart:
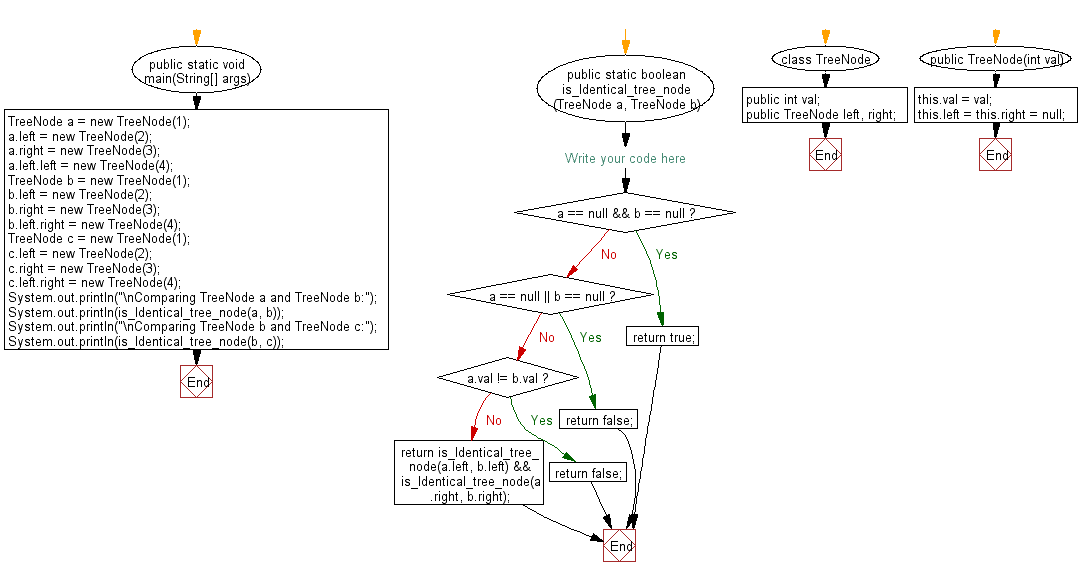
Java Code Editor:
Company: Bloomberg
Contribute your code and comments through Disqus.
Previous: Write a Java program to find the length of last word of a given string. The string contains upper/lower-case alphabets and empty space characters ' '.
Next: Write a Java program to accept a positive number and repeatedly add all its digits until the result has only one digit.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics