Java: Print a face
Java Basic: Exercise-16 with Solution
Write a Java program to print a face.
Pictorial Presentation:
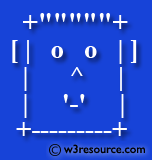
Sample Solution:
Java Code:
public class Exercise16 {
public static void main(String[] args)
{
// Display a pattern to create an ASCII art representation of a simple face
System.out.println(" +\"\"\"\"\"+ ");
System.out.println("[| o o |]");
System.out.println(" | ^ | ");
System.out.println(" | '-' | ");
System.out.println(" +-----+ ");
}
}
Sample Output:
+"""""+ [| o o |] | ^ | | '-' | +-----+
Flowchart:
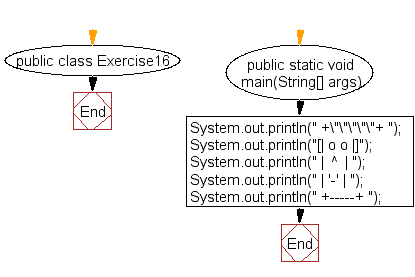
Sample Solution (using array):
Java Code:
public class Main {
public static void main(String[] args) {
// Create an array to store lines of an ASCII art representation
String[] arra = new String[5];
// Populate the array with lines to form an ASCII art representation of a simple face
arra[0] = " +\"\"\"\"\"+ ";
arra[1] = "[| o o |]";
arra[2] = " | ^ |";
arra[3] = " | '-' |";
arra[4] = " +-----+";
// Use a loop to print each line of the ASCII art representation
for (int i = 0; i < 5; i++) {
System.out.println(arra[i]);
}
}
}
Flowchart:
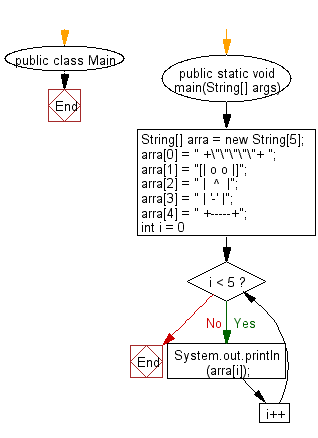
Java Code Editor:
Previous: Write a Java program to swap two variables.
Next: Write a Java program to add two binary numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics