Java: Returns the largest integer but not larger than the base-2 logarithm of a specified integer
Largest Integer Base-2 Logarithm
Write a Java program that returns the largest integer but not larger than the base-2 logarithm of a given integer.
Original Number: 2350
Result: 11
Sample Solution:
Java Code:
import java.util.Scanner;
public class Solution {
public static void main(String[] args) {
// Initializing an integer variable 'n' with the value 2350
int n = 2350;
// Displaying the original number
System.out.printf("Original Number: %d\n", n);
// Initializing a variable to count the number of right shifts
int shift_right_count = 0;
// Performing right shift operations until 'n' becomes zero
do {
n >>= 1; // Right shifting 'n' by 1 bit
shift_right_count++; // Incrementing the shift count
} while (n != 0); // Loop continues until 'n' becomes zero
shift_right_count--; // Decrementing the shift count by 1 to correct the count
// Displaying the final result (shift count)
System.out.printf("Result: %s\r\n", shift_right_count);
}
}
Sample Output:
Original Number: 2350 Result: 11
Flowchart:
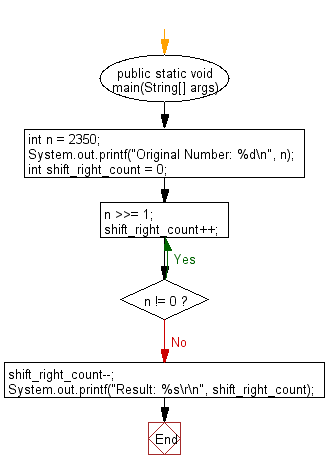
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Java program to print an array after changing the rows and columns of a given two-dimensional array.
Next: Write a Java program to prove that Euclid’s algorithm computes the greatest common divisor of two positive given integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics