Java: Accepts two double variables and test the specified condition
Java Basic: Exercise-153 with Solution
Write a Java program that accepts two double variables and test if both strictly between 0 and 1 and false otherwise.
Sample Solution:
Java Code:
import java.util.Scanner;
public class Solution {
public static void main(String[] args) {
// Creating a Scanner object to take input from the user
Scanner in = new Scanner(System.in);
// Prompting the user to input the first number
System.out.print("Input first number: ");
double n1 = in.nextDouble();
// Prompting the user to input the second number
System.out.print("Input second number: ");
double n2 = in.nextDouble();
// Checking if both numbers are greater than 0 and less than 1
System.out.println(n1 > 0 && n1 < 1 && n2 > 0 && n2 < 1);
}
}
Sample Output:
Input first number: 1.25 Input second number: 2.15 false
Input first number: .55 Input second number: .75 true
Flowchart:
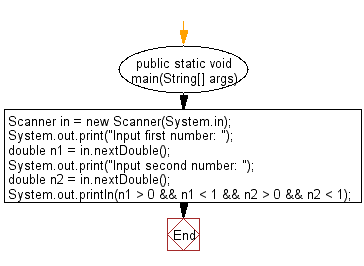
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Java program that accepts four integer from the user and prints equal if all four are equal, and not equal otherwise.
Next: Write a Java program to print the contents of a two-dimensional Boolean array where t will represent true and f will represent false.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/java-exercises/basic/java-basic-exercise-153.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics