Java: Check if two given strings are anagrams or not
Java Basic: Exercise-142 with Solution
Write a Java program to check if two strings are anagrams or not.
According to wikipedia "An anagram is a word or phrase formed by rearranging the letters of a different word or phrase, typically using all the original letters exactly once. For example, the word anagram can be rearranged into nag a ram, or the word binary into brainy."
Pictorial Presentation:
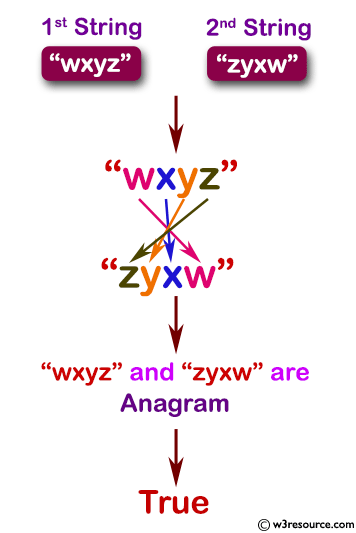
Sample Solution:
Java Code:
import java.util.*
public class Solution {
/**
* @param s: The first string
* @param b: The second string
* @return true or false
*/
public static boolean anagram_test(String str1, String str2) {
// Check if either input string is null
if (str1 == null || str2 == null) {
return false;
}
// Check if the lengths of the two strings are different
else if (str1.length() != str2.length()) {
return false;
}
// Check if both strings are empty (an edge case)
else if (str1.length() == 0 && str2.length() == 0) {
return true;
}
// Create an integer array to count character occurrences
int[] count = new int[256];
// Count character occurrences in both strings
for (int i = 0; i < str1.length(); i++) {
count[str1.charAt(i)]++;
count[str2.charAt(i)]--;
}
// Check if all counts in the array are zero, indicating anagrams
for (int num : count) {
if (num != 0) {
return false;
}
}
// If all checks pass, the strings are anagrams
return true;
}
public static void main(String[] args) {
// Test case: Check if the strings "wxyz" and "zyxw" are anagrams
String str1 = "wxyz";
String str2 = "zyxw";
// Print the original strings
System.out.println("String-1 : " + str1);
System.out.println("String-2 : " + str2);
// Check if the two given strings are anagrams and print the result
System.out.println("Check if two given strings are anagrams or not?: " + anagram_test(str1, str2));
}
}
Sample Output:
String-1 : wxyz String-2 : zyxw Check if two given strings are anagrams or not?: true
Flowchart:
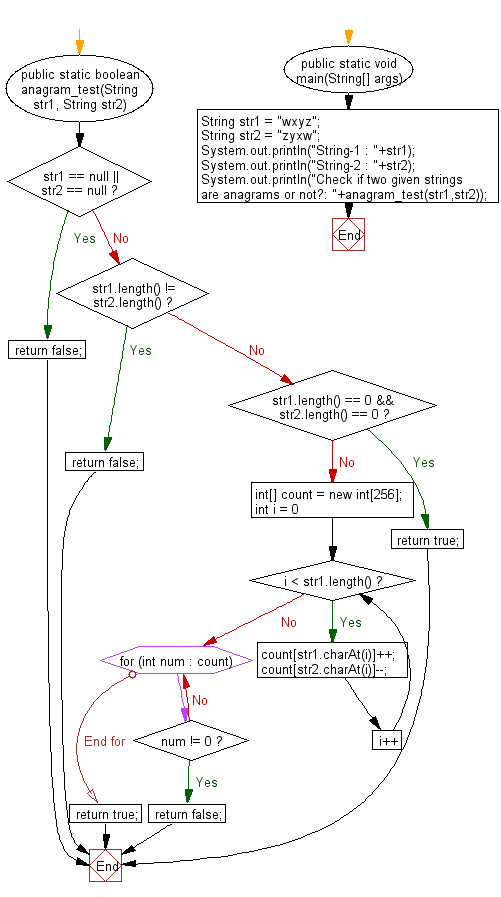
Java Code Editor:
Previous: Write a Java program to check if a given string has all unique characters.
Next: Write a Java program to merge two given sorted lists.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/java-exercises/basic/java-basic-exercise-142.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics