Java: Find the distinct ways you can climb to the top
Distinct Ways to Climb Stairs
Write a Java program to find distinct ways to climb to the top (n steps to reach the top) of stairs. Each time you climb, you can climb 1 or 2 steps.
Example: n = 5 a) 1+1+1+1+1 = 5 b) 1+1+1+2 = 5 c) 1+2+2 = 5 d) 2+2+1 = 5 e) 2+1+1+1 = 5 f) 2+1+2 = 5 g) 1+2+1+1 = 5 h) 1+1+2+1 = 5
Pictorial Presentation:
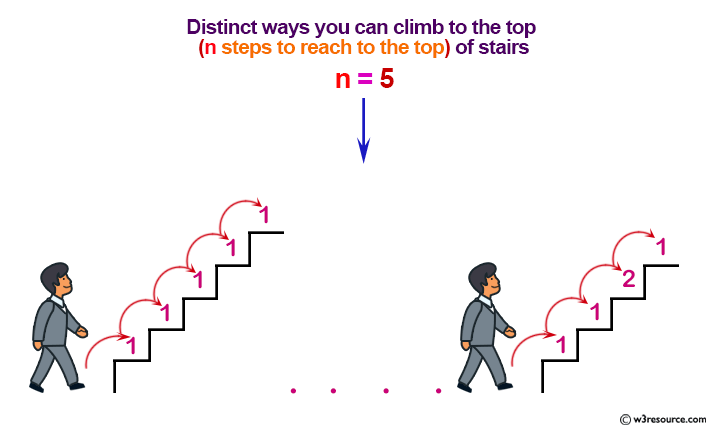
Sample Solution:
Java Code:
import java.util.*
class Solution {
// Static method to calculate the distinct ways to climb stairs
public static int climbStairs(int n) {
if (n <= 1) {
return 1; // If there is 0 or 1 step, there is only 1 way to climb.
}
int[] s_case = new int[n + 1]; // Create an array to store the number of distinct ways for each step count.
s_case[0] = 1; // There is 1 way to climb 0 steps.
s_case[1] = 1; // There is 1 way to climb 1 step.
for (int i = 2; i <= n; i++) {
// Calculate the number of distinct ways for each step by adding the ways from the previous two steps.
s_case[i] = s_case[i - 1] + s_case[i - 2];
}
return s_case[n]; // Return the number of distinct ways to climb n steps.
}
public static void main(String[] args) {
int steps = 5; // The number of steps to climb
System.out.println("Distinct ways can you climb to the top: " + climbStairs(steps));
}
}
Sample Output:
Distinct ways can you climb to the top: 8
Flowchart:
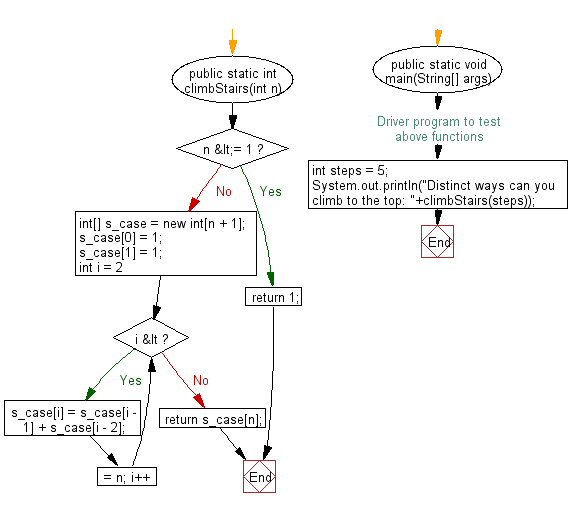
Java Code Editor:
Previous:Write a Java program to find a path from top left to bottom in right direction which minimizes the sum of all numbers along its path.
Next: Write a Java program to remove duplicates from a sorted linked list
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics