Java: Find the new length of a given sorted array where each element appear only once
Java Basic: Exercise-131 with Solution
Write a Java program to find the updated length of a sorted array where each element appears only once (remove duplicates).
Pictorial Presentation:
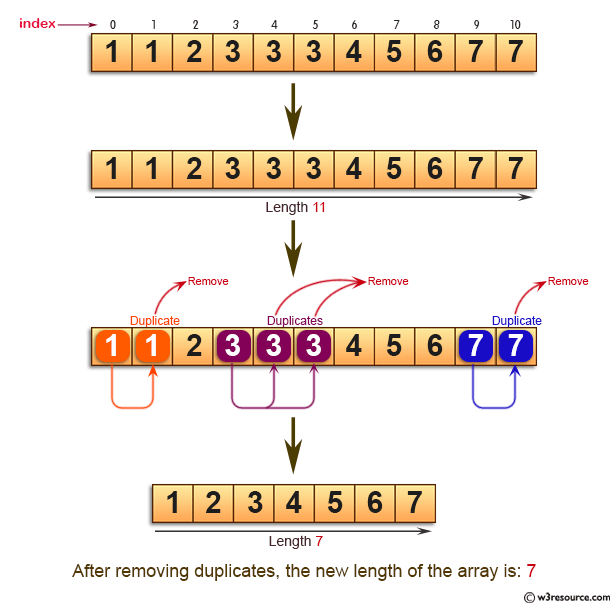
Sample Solution:
Java Code:
import java.util.Arrays;
class Solution {
// Static method to remove duplicates from the given array
static int removeDuplicates(int[] nums) {
if (nums == null) {
return 0;
}
if (nums.length <= 1) {
return nums.length;
}
int current_pos = 0;
int moving_pos;
for (moving_pos = 1; moving_pos < nums.length; moving_pos++) {
// Check if the current element is different from the next element
if (nums[current_pos] != nums[moving_pos]) {
// If different, move the unique element to the next position in the array
nums[current_pos + 1] = nums[moving_pos];
current_pos++; // Increment the position for the unique element
}
}
// The new length of the array is one more than the current position
return current_pos + 1;
}
/* Driver program to test above functions */
public static void main(String[] args) {
int[] nums = {1, 1, 2, 3, 3, 3, 4, 5, 6, 7, 7};
System.out.println("Original array: " + Arrays.toString(nums));
System.out.println("The length of the original array is: " + nums.length);
System.out.println("After removing duplicates, the new length of the array is: " + removeDuplicates(nums));
}
}
Sample Output:
Original array: [1, 1, 2, 3, 3, 3, 4, 5, 6, 7, 7] The length of the original array is: 11 After removing duplicates, the new length of the array is: 7
Flowchart:
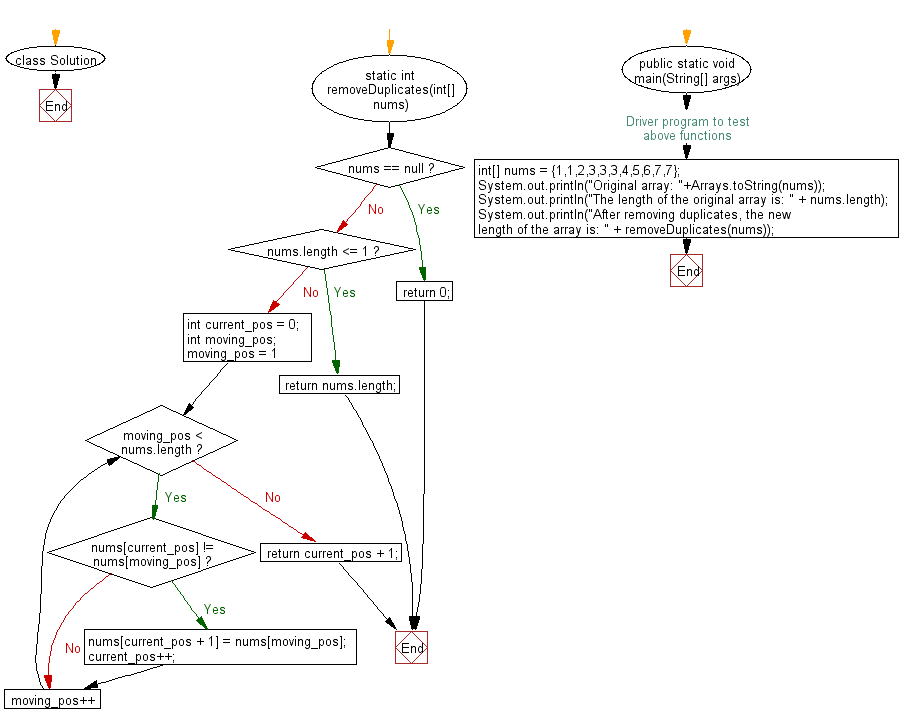
Java Code Editor:
Previous: Write a Java program to find the maximum depth of a given a binary tree.
Next: Write a Java program to find the new length of a given sorted array where duplicate elements appeared at most twice.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics