Java: Reverse a given linked list
Reverse Linked List
Write a Java program to reverse a linked list.
Example: For linked list 20->40->60->80, the reversed linked list is 80->60->40->20
Pictorial Presentation:
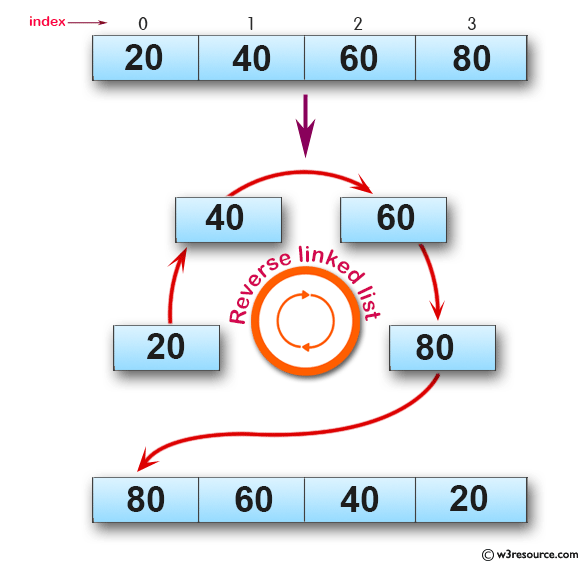
Sample Solution:
Java Code:
class LinkedList {
// Static variable to store the head of the linked list
static Node head;
static class Node {
int data; // Data stored in the node
Node next_node; // Reference to the next node
Node(int d) {
data = d;
next_node = null;
}
}
// Function to reverse the linked list
Node reverse(Node node) {
Node prev_node = null;
Node current_node = node;
Node next_node = null;
while (current_node != null) {
next_node = current_node.next_node;
current_node.next_node = prev_node;
prev_node = current_node;
current_node = next_node;
}
node = prev_node;
return node;
}
// Function to print the elements of the linked list
void printList(Node node) {
while (node != null) {
System.out.print(node.data + " ");
node = node.next_node;
}
}
public static void main(String[] args) {
LinkedList list = new LinkedList();
// Create a linked list with some initial values
list.head = new Node(20);
list.head.next_node = new Node(40);
list.head.next_node.next_node = new Node(60);
list.head.next_node.next_node.next_node = new Node(80);
// Print the original linked list
System.out.println("Original Linked list:");
list.printList(head);
// Reverse the linked list
head = list.reverse(head);
System.out.println("");
// Print the reversed linked list
System.out.println("Reversed Linked list:");
list.printList(head);
}
}
Sample Output:
Original Linked list: 20 40 60 80 Reversed Linked list: 80 60 40 20
Flowchart:
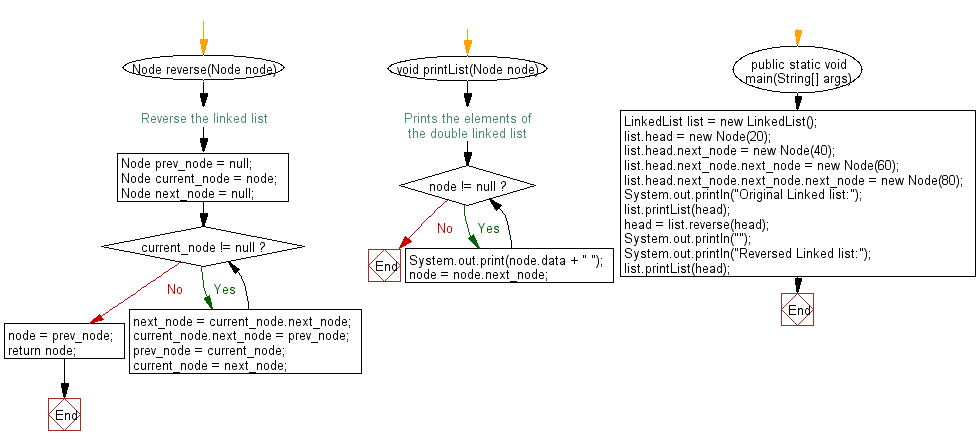
Java Code Editor:
Previous: Write a Java program that searches a value in an m x n matrix.
Next: Write a Java program to find a contiguous subarray with largest sum from a given array of integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics