Java: Calculate and print the average of five numbers
Average of Three Numbers
Write a Java program that takes five numbers as input to calculate and print the average of the numbers.
Test Data:
Input first number: 10
Input second number: 20
Input third number: 30
Input fourth number: 40
Enter fifth number: 50
Pictorial Presentation:
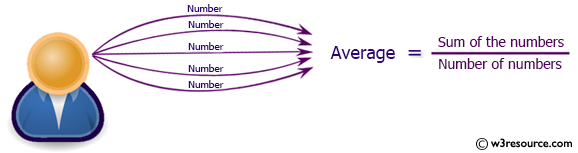
Sample Solution-1
Java Code:
import java.util.Scanner;
public class Exercise12 {
public static void main(String[] args) {
// Create a Scanner object to read input from the user
Scanner in = new Scanner(System.in);
// Prompt the user to input the first number
System.out.print("Input first number: ");
// Read and store the first number
int num1 = in.nextInt();
// Prompt the user to input the second number
System.out.print("Input second number: ");
// Read and store the second number
int num2 = in.nextInt();
// Prompt the user to input the third number
System.out.print("Input third number: ");
// Read and store the third number
int num3 = in.nextInt();
// Prompt the user to input the fourth number
System.out.print("Input fourth number: ");
// Read and store the fourth number
int num4 = in.nextInt();
// Prompt the user to input the fifth number
System.out.print("Enter fifth number: ");
// Read and store the fifth number
int num5 = in.nextInt();
// Calculate and print the average of the five numbers
System.out.println("Average of five numbers is: " + (num1 + num2 + num3 + num4 + num5) / 5);
}
}
Explanation:
In the exercise above -
- It prompts the user to input five different numbers, one by one.
- It reads and stores each number in separate variables: num1, num2, num3, num4, and num5.
- It calculates the average of these five numbers by adding them together and dividing the sum by 5.
- It displays the calculated average with the message "Average of five numbers is:" to the console.
Sample Output:
Input first number: 10 Input second number: 20 Input third number: 30 Input fourth number: 40 Enter fifth number: 50 Average of five numbers is: 30
Flowchart:
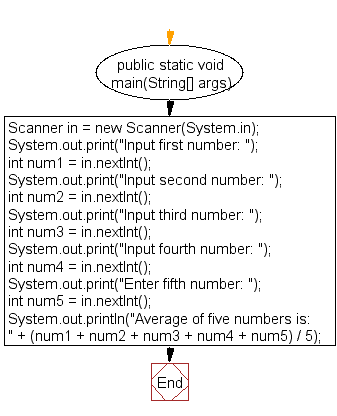
Sample Solution-2
Java Code:
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
// Initialize variables for sum and counting
double num = 0;
double x = 1;
// Create a Scanner object to read input from the user
Scanner sc = new Scanner(System.in);
// Prompt the user to input the number (n) for which to calculate the average
System.out.println("Input the number(n) you want to calculate the average: ");
int n = sc.nextInt();
// Use a loop to collect n numbers and calculate their sum
while (x <= n) {
System.out.println("Input number " + "(" + (int) x + ")" + ":");
num += sc.nextInt();
x += 1;
}
// Calculate the average of the collected numbers
double avgn = (num / n);
// Display the calculated average
System.out.println("Average: " + avgn);
}
}
Sample Output:
Input the number(n) you want to calculate the average: 4 Input number (1): 2 Input number (2): 4 Input number (3): 4 Input number (4): 2 Average:3.0
Flowchart:
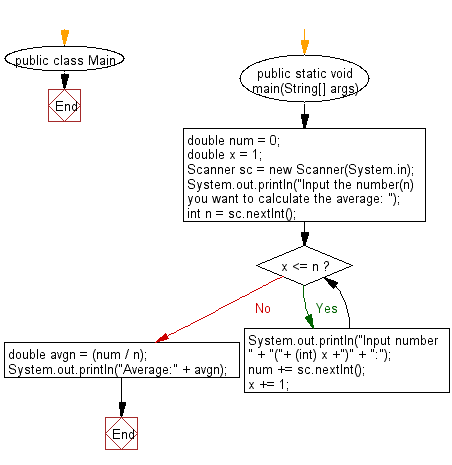
Java Code Editor:
Previous: Write a Java program to print the area and perimeter of a circle.
Next: Write a Java program to print the area and perimeter of a rectangle.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics