Java: Iterating on two integers to get Fizz, Buzz and FizzBuzz
Java Basic: Exercise-116 with Solution
Write a Java program that iterates integers from 1 to 100. For multiples of three print "Fizz" instead of the number and print "Buzz" for five. When the number is divided by three and five, print "fizz buzz".
Sample Solution:
Java Code:
import java.util.*;
public class Exercise116 {
public static void main(String[] args) {
// Iterate from 1 to 100
for (int i = 1; i <= 100; i++) {
if (i % 3 == 0 && i % 5 == 0) {
// Check if the number is divisible by both 3 and 5 (fizz buzz)
System.out.printf("\n%d: fizz buzz", i);
} else if (i % 5 == 0) {
// Check if the number is divisible by 5 (buzz)
System.out.printf("\n%d: buzz", i);
} else if (i % 3 == 0) {
// Check if the number is divisible by 3 (fizz)
System.out.printf("\n%d: fizz", i);
}
}
System.out.printf("\n");
}
}
Sample Output:
3: fizz 5: buzz 6: fizz 9: fizz 10: buzz 12: fizz 15: fizz buzz 18: fizz 20: buzz 21: fizz 24: fizz 25: buzz 27: fizz 30: fizz buzz 33: fizz 35: buzz 36: fizz 39: fizz 40: buzz 42: fizz 45: fizz buzz 48: fizz 48: fizz 50: buzz 51: fizz 54: fizz 55: buzz 57: fizz 60: fizz buzz 63: fizz 65: buzz 66: fizz 69: fizz 70: buzz 72: fizz 75: fizz buzz 78: fizz 80: buzz 81: fizz 84: fizz 85: buzz 87: fizz 90: fizz buzz 93: fizz 95: buzz 96: fizz 99: fizz 100: buzz
Flowchart:
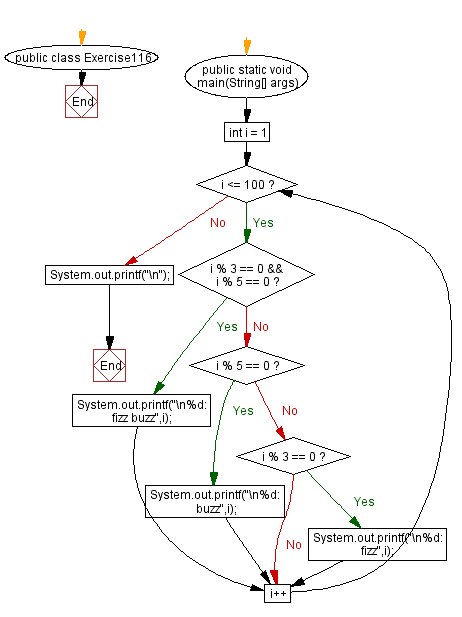
Java Code Editor:
Previous: Write a Java program to check if a positive number is a palindrome or not.
Next: Write a Java program to compute the square root of an given integer.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/java-exercises/basic/java-basic-exercise-116.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics