Java: Form a staircase shape of n coins where every k-th row must have exactly k coins
Java Basic: Exercise-109 with Solution
Write a Java program to form a staircase shape of n coins where every k-th row must have exactly k coins.
Example 1:
n = 3
The coins can form the following rows:
$
$ $
We will return 2 rows.
Example 2:
n = 4
The coins can form the following rows:
$
$ $
$
We will return 2 rows as the 3rd row is incomplete.
Example 3:
n = 5
The coins can form the following rows:
$
$ $
$ $
We will return 2 rows as the 3rd row is incomplete.
Sample Solution:
Java Code:
import java.util.Scanner;
public class Example109 {
public static void main(String[] arg) {
// Create a Scanner object for user input
Scanner in = new Scanner(System.in);
// Prompt the user to input a positive integer
System.out.print("Input a positive integer: ");
// Read the user's input as an integer
int n = in.nextInt();
if (n > 0) {
// Check if n is a positive integer
double sqrtResult = Math.sqrt(8 * (long) n + 1); // Calculate the square root
int numRows = (int) ((sqrtResult - 1) / 2); // Calculate the number of rows
System.out.println("Number of rows: " + numRows);
}
// Close the input scanner
in.close();
}
}
Sample Output:
Input a positive integer: 5 Number of rows: 2
Flowchart:
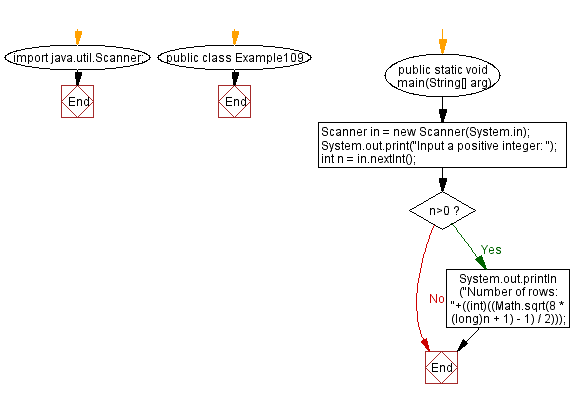
Java Code Editor:
Previous: Write a Java program to add all the digits of a given positive integer until the result has a single digit.
Next: Write a Java program to check whether an given integer is a power of 4 or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/java-exercises/basic/java-basic-exercise-109.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics