Java: Cyclically rotate a given array clockwise by one
Java Array: Exercise-45 with Solution
Write a Java program to cyclically rotate a given array clockwise by one.
Pictorial Presentation:
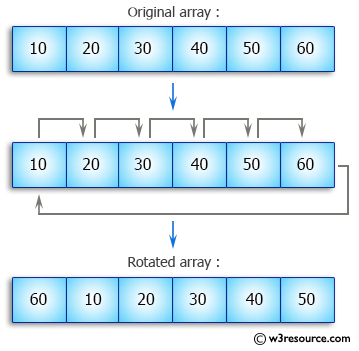
Sample Solution:
Java Code:
// Import the Arrays class to use the toString method for array display.
import java.util.Arrays;
// Define the Main class.
public class Main
{
// Define an integer array named 'arra'.
static int arra[] = new int[]{10, 20, 30, 40, 50, 60};
// Define a method to rotate the array elements.
static void rotate_array()
{
// Store the last element of the array in variable 'a'.
int a = arra[arra.length - 1];
int i;
// Iterate through the array to shift elements to the right.
for (i = arra.length - 1; i > 0; i--)
arra[i] = arra[i - 1];
// Set the first element of the array to 'a' (rotating it to the end).
arra[0] = a;
}
// The main method for executing the program.
public static void main(String[] args)
{
// Display the original array.
System.out.println("Original array:");
System.out.println(Arrays.toString(arra));
// Call the rotate_array method to rotate the array.
rotate_array();
// Display the rotated array.
System.out.println("Rotated array:");
System.out.println(Arrays.toString(arra));
}
}
Sample Output:
Original arraay: [10, 20, 30, 40, 50, 60] Rotated arraay: [60, 10, 20, 30, 40, 50]
Flowchart:
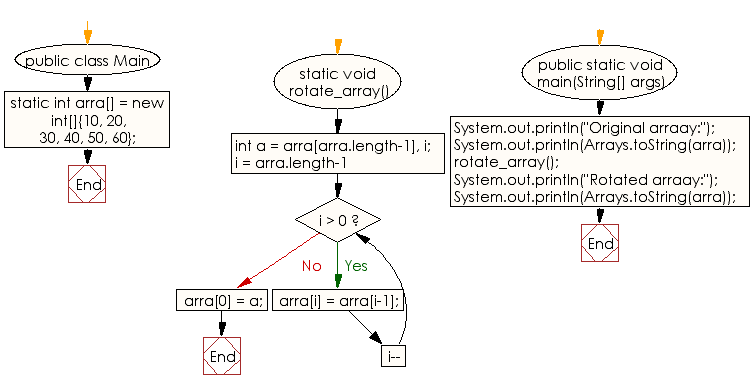
Java Code Editor:
Previous: Write a Java program to count the number of possible triangles from a given unsorted array of positive integers.
Next: Write a Java program to check whether there is a pair with a specified sum of a given sorted and rotated array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/java-exercises/array/java-array-exercise-45.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics