Java: Find a missing number in an array
Write a Java program to find a missing number in an array.
Pictorial Presentation:
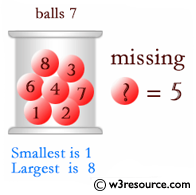
Sample Solution:
Java Code:
// Import the java.util package to use utility classes, including Scanner.
import java.util.*;
// Define a class named Exercise24.
public class Exercise24 {
// The main method for executing the program.
public static void main(String[] args) {
// Declare variables for total number and an array of integers.
int total_num;
int[] numbers = new int[]{1, 2, 3, 4, 6, 7};
// Assign the value 7 to the variable total_num.
total_num = 7;
// Calculate the expected sum of numbers using the arithmetic sum formula.
int expected_num_sum = total_num * ((total_num + 1) / 2);
// Initialize a variable to store the sum of numbers.
int num_sum = 0;
// Iterate through the elements of the 'numbers' array.
for (int i : numbers) {
// Add each element to the 'num_sum' variable.
num_sum += i;
}
// Calculate the missing number by subtracting 'num_sum' from 'expected_num_sum'.
System.out.print(expected_num_sum - num_sum);
// Print a newline character to end the line.
System.out.print("\n");
}
}
Sample Data: 1,2,3,4,6,7
Sample Output:
5
Flowchart:
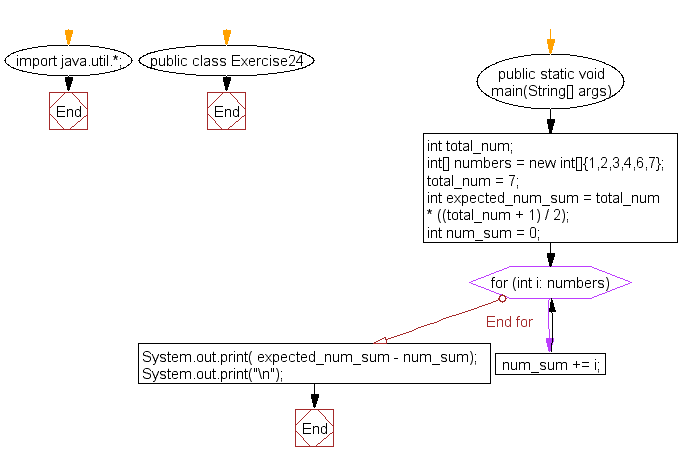
Java Code Editor:
Previous: Write a Java program to test the equality of two arrays.
Next: Write a Java program to find common elements from three sorted (in non-decreasing order) arrays.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics