Java: Find all Pairs of elements in an array whose sum is equal to a specified number
Java Array: Exercise-22 with Solution
Write a Java program to find all pairs of elements in an array whose sum is equal to a specified number.
Pictorial Presentation:
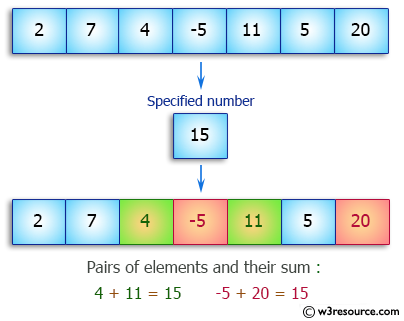
Sample Solution:
Java Code :
// Define a class named Exercise22.
public class Exercise22 {
// Create a static method named pairs_value that takes an integer array and an input number.
static void pairs_value(int inputArray[], int inputNumber) {
System.out.println("Pairs of elements and their sum : ");
// Iterate through the elements of the inputArray.
for (int i = 0; i < inputArray.length; i++) {
// Iterate through the elements following the current i.
for (int j = i + 1; j < inputArray.length; j++) {
// Check if the sum of inputArray[i] and inputArray[j] equals the inputNumber.
if (inputArray[i] + inputArray[j] == inputNumber) {
// Print the pair of elements and their sum.
System.out.println(inputArray[i] + " + " + inputArray[j] + " = " + inputNumber);
}
}
}
}
// The main method for executing the program.
public static void main(String[] args) {
// Call the pairs_value method with a sample array and input number.
pairs_value(new int[]{2, 7, 4, -5, 11, 5, 20}, 15);
// Call the pairs_value method with another sample array and input number.
pairs_value(new int[]{14, -15, 9, 16, 25, 45, 12, 8}, 30);
}
}
Sample Output:
Pairs of elements and their sum : 4 + 11 = 15 -5 + 20 = 15 Pairs of elements and their sum : 14 + 16 = 30 -15 + 45 = 30
Flowchart:
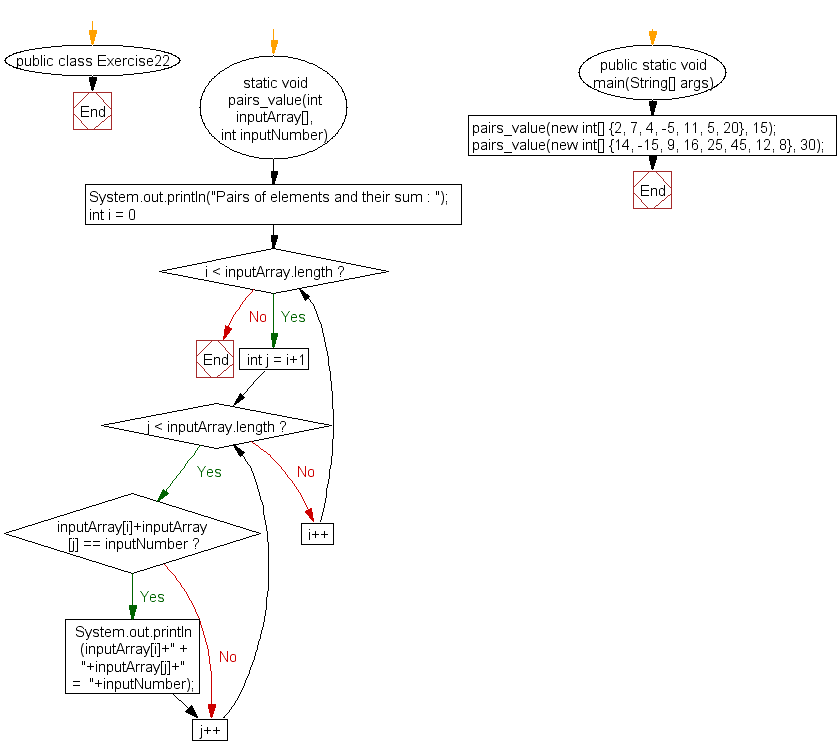
Java Code Editor:
Previous: Write a Java program to convert an ArrayList to an array.
Next: Write a Java program to test the equality of two arrays.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/java-exercises/array/java-array-exercise-22.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics