Java: Sort a numeric array and a string array
Java Array: Exercise-1 with Solution
Write a Java program to sort a numeric array and a string array.
Pictorial Presentation:
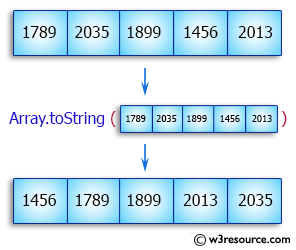
Sample Solution:
Java Code:
// Import the necessary class from the Java utility library.
import java.util.Arrays;
// Define a class named Exercise1.
public class Exercise1 {
// The main method where the program execution starts.
public static void main(String[] args) {
// Declare and initialize an integer array.
int[] my_array1 = {
1789, 2035, 1899, 1456, 2013,
1458, 2458, 1254, 1472, 2365,
1456, 2165, 1457, 2456
};
// Declare and initialize a string array.
String[] my_array2 = {
"Java",
"Python",
"PHP",
"C#",
"C Programming",
"C++"
};
// Print the original numeric array.
System.out.println("Original numeric array : " + Arrays.toString(my_array1));
// Sort the numeric array in ascending order.
Arrays.sort(my_array1);
// Print the sorted numeric array.
System.out.println("Sorted numeric array : " + Arrays.toString(my_array1));
// Print the original string array.
System.out.println("Original string array : " + Arrays.toString(my_array2));
// Sort the string array in lexicographical (alphabetical) order.
Arrays.sort(my_array2);
// Print the sorted string array.
System.out.println("Sorted string array : " + Arrays.toString(my_array2));
}
}
Sample Output:
Original numeric array : [1789, 2035, 1899, 1456, 2013, 1458, 2458, 1254, 1472, 2365, 1456, 2165, 1457, 2456] Sorted numeric array : [1254, 1456, 1456, 1457, 1458, 1472, 1789, 1899, 2013, 2035, 2165, 2365, 2456, 2458] Original string array : [Java, Python, PHP, C#, C Programming, C++] Sorted string array : [C Programming, C#, C++, Java, PHP, Python]
Flowchart:
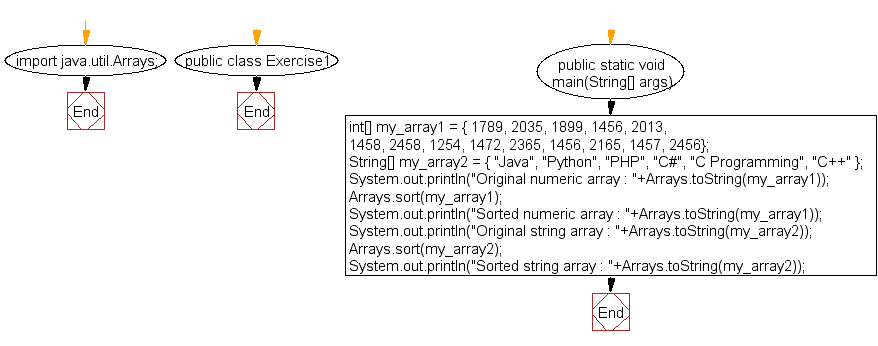
Java Code Editor:
Previous: Java Array Exercises
Next: Write a Java program to sum values of an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/java-exercises/array/java-array-exercise-1.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics