C#: Declares a structure with a property, a method, and a private field
Write a program in C# Sharp that declares a struct with a property, a method, and a private field.
Sample Solution:-
C# Sharp Code:
using System;
// Declaration of a structure named newStruct
struct newStruct
{
private int num; // Private field 'num' in the structure
// Public property 'n' with getter and setter
public int n
{
// Getter returns the value of 'num'
get
{
return num;
}
// Setter assigns value to 'num' if it is less than 50
set
{
if (value < 50)
num = value;
}
}
// Method to display the value stored in 'num'
public void clsMethod()
{
Console.WriteLine("\nThe stored value is: {0}\n", num);
}
}
// Main class
class strucExer6
{
// Main method
public static void Main()
{
// Displaying a message indicating the structure's components
Console.Write("\n\nDeclares a structure with a property, a method, and a private field :\n");
Console.Write("----------------------------------------------------------------------\n");
// Creating an instance of newStruct
newStruct myInstance = new newStruct();
// Assigning a value using the property 'n'
myInstance.n = 15;
// Calling the method 'clsMethod' to display the stored value
myInstance.clsMethod();
}
}
Sample Output:
Declares a structure with a property, a method, and a private field : ---------------------------------------------------------------------- The stored value is: 15
Flowchart:
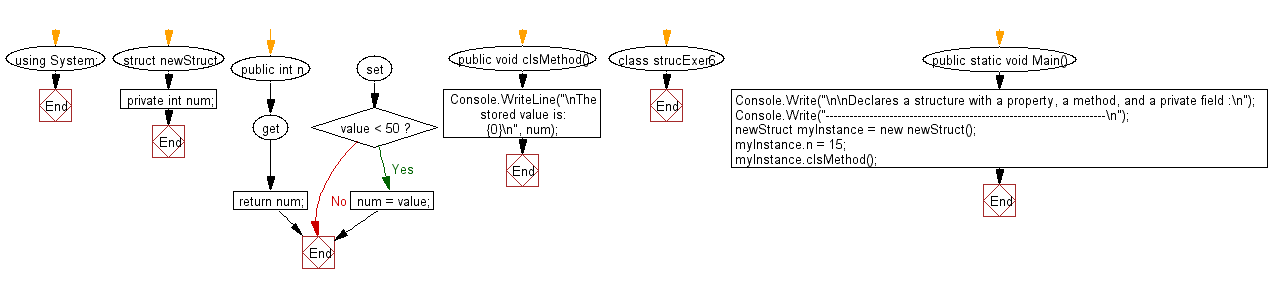
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to show what happen when a struct and a class instance is passed to a method.
Next: Write a program in C# Sharp to demonstrates struct initialization using both default and parameterized constructors.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.