C#: Method that returns a structure
Write a program in C# Sharp to implement a method that returns a structure. This includes calling the method and using its value.
Sample Solution:-
C# Sharp Code:
using System;
// Struct defining a sample structure
public struct sampStru
{
private double val; // Private field to store a double value
// Property 'Value' to get and set the private 'val' field
public double Value
{
get { return val; }
set { val = value; }
}
// Method to read a double value from the console
public double Read()
{
return double.Parse(Console.ReadLine());
}
}
// Struct defining a square using sampStru structure
public struct Square
{
sampStru ln; // Instance of sampStru for length
sampStru ht; // Instance of sampStru for height
// Property 'Length' to get and set the length of the square
public sampStru Length
{
get { return ln; }
set { ln = value; }
}
// Property 'Breadth' to get and set the breadth of the square
public sampStru Breadth
{
get { return ht; }
set { ht = value; }
}
// Method to create a new square by taking user input for dimensions
public void newSquare()
{
sampStru rct = new sampStru();
Console.WriteLine("\nInput the dimensions of the Square (equal length and breadth): ");
ln = sqrLength();
Console.Write("breadth : ");
ht.Value = rct.Read();
}
// Method to input the length of the square
public sampStru sqrLength()
{
sampStru rct = new sampStru();
Console.Write("length : ");
rct.Value = rct.Read();
return rct;
}
}
// Main class
public class strucExer10
{
// Main method
static void Main()
{
Console.Write("\n\nMethod that returns a structure :\n");
Console.Write("--------------------------------------\n");
// Create an instance of Square
var Sqre = new Square();
// Invoke the method to create a new square by taking user input
Sqre.newSquare();
Console.WriteLine(); // Blank line for readability
Console.WriteLine("Perimeter and Area of the square:");
Console.WriteLine("Length: {0}", Sqre.Length.Value); // Display length
Console.WriteLine("Breadth: {0}", Sqre.Breadth.Value); // Display breadth
Console.WriteLine("Perimeter: {0}", (Sqre.Length.Value + Sqre.Breadth.Value) * 2); // Calculate and display perimeter
Console.WriteLine("Area: {0}\n", Sqre.Length.Value * Sqre.Breadth.Value); // Calculate and display area
}
}
Sample Output:
Method that returns a structure : -------------------------------------- Input the dimensions of the Square( equal length and breadth ) : length : 10 breadth : 20 Perimeter and Area of the square : Length: 10 Breadth: 20 Perimeter: 60 Area: 200
Flowchart:
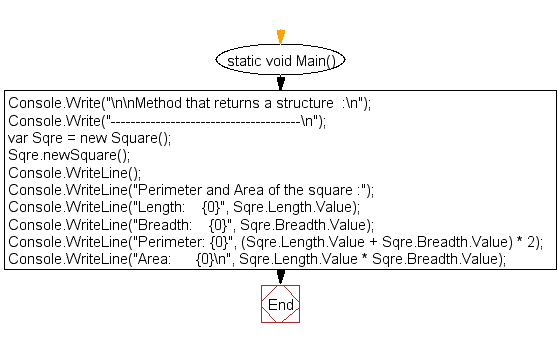
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to insert the information of two books.
Next: C# Sharp DateTime Exercises.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics