C#: Declare a simple structure
Write a program in C# Sharp to declare a simple structure.
Sample Solution:-
C# Sharp Code:
Sample Output:
Declare a simple structure : -------------------------------- The sum of x and y is 40
Flowchart:
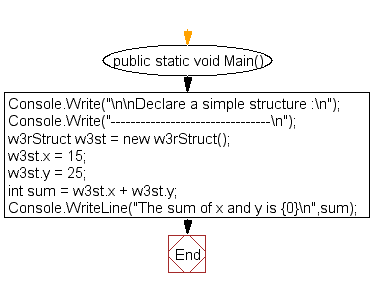
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: C# Sharp STRUCTURE Exercises with solution.
Next: Write a program in C# Sharp to declare a simple structure and use of static fields inside a struct.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.