C#: Copy one string into another string
Write a program in C# Sharp to copy one string to another string.
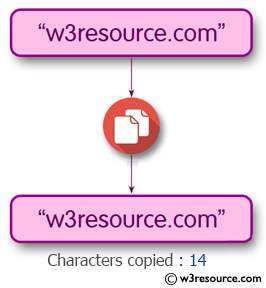
Sample Solution:-
C# Sharp Code:
using System;
// Define the Exercise8 class
public class Exercise8
{
// Main method - entry point of the program
public static void Main()
{
string str1; // Declare a string variable to hold user input
int i, l; // Declare variables for iteration and length
// Prompt the user to input a string
Console.Write("\n\nCopy one string into another string:\n");
Console.Write("-----------------------------------------\n");
Console.Write("Input the string: ");
str1 = Console.ReadLine(); // Read the input string from the user
l = str1.Length; // Get the length of the input string
string[] str2 = new string[l]; // Initialize an array to hold characters from the string
// Copy each character from str1 to str2 character by character
i = 0; // Initialize the iteration variable
while (i < l)
{
string tmp = str1[i].ToString(); // Convert the character to a string
str2[i] = tmp; // Copy the character to the str2 array
i++; // Move to the next character
}
// Display the original string (str1), copied string (str2), and the number of characters copied
Console.Write("\nThe First string is: {0}\n", str1);
Console.Write("The Second string is: {0}\n", string.Join("", str2)); // Join characters in str2 to form a string
Console.Write("Number of characters copied: {0}\n\n", i); // Display the number of characters copied
}
}
Sample Output:
Copy one string into another string : ----------------------------------------- Input the string : w3resource.com The First string is : w3resource.com The Second string is : w3resource.com Number of characters copied : 14
Flowchart:
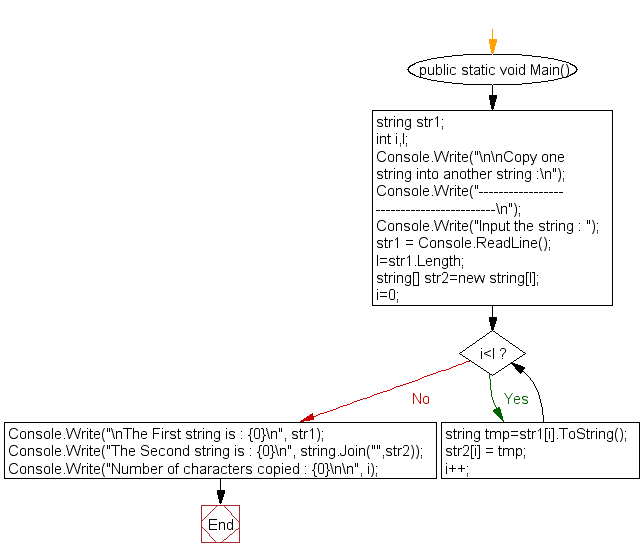
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to count a total number of alphabets, digits and special characters in a string.
Next: Write a program in C# Sharp to count a total number of vowel or consonant in a string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.