C#: Count a total number of alphabets, digits and special characters
Write a program in C# Sharp to count the number of alphabets, digits and special characters in a string.
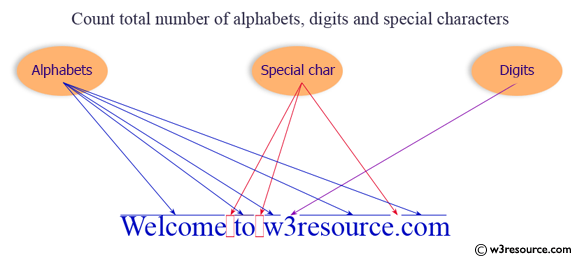
Sample Solution:-
C# Sharp Code:
using System;
// Define the Exercise7 class
public class Exercise7
{
// Main method - entry point of the program
public static void Main()
{
string str; // Declare a string variable to hold user input
int alp, digit, splch, i, l; // Declare variables to count alphabets, digits, and special characters
alp = digit = splch = i = 0; // Initialize count variables to zero
// Prompt the user to input a string
Console.Write("\n\nCount total number of alphabets, digits, and special characters:\n");
Console.Write("--------------------------------------------------------------------\n");
Console.Write("Input the string: ");
str = Console.ReadLine(); // Read the input string from the user
l = str.Length; // Get the length of the input string
// Loop through each character in the string to count alphabets, digits, and special characters
while (i < l)
{
if ((str[i] >= 'a' && str[i] <= 'z') || (str[i] >= 'A' && str[i] <= 'Z'))
{
alp++; // Increment the alphabet count if the character is an alphabet
}
else if (str[i] >= '0' && str[i] <= '9')
{
digit++; // Increment the digit count if the character is a digit
}
else
{
splch++; // Increment the special character count for all other characters
}
i++; // Move to the next character
}
// Display the counts of alphabets, digits, and special characters in the string
Console.Write("Number of Alphabets in the string is: {0}\n", alp);
Console.Write("Number of Digits in the string is: {0}\n", digit);
Console.Write("Number of Special characters in the string is: {0}\n\n", splch);
}
}
Sample Output:
Count total number of alphabets, digits and special characters : -------------------------------------------------------------------- Input the string : Welcome to w3resource.com Number of Alphabets in the string is : 21 Number of Digits in the string is : 1 Number of Special characters in the string is : 3
Flowchart:
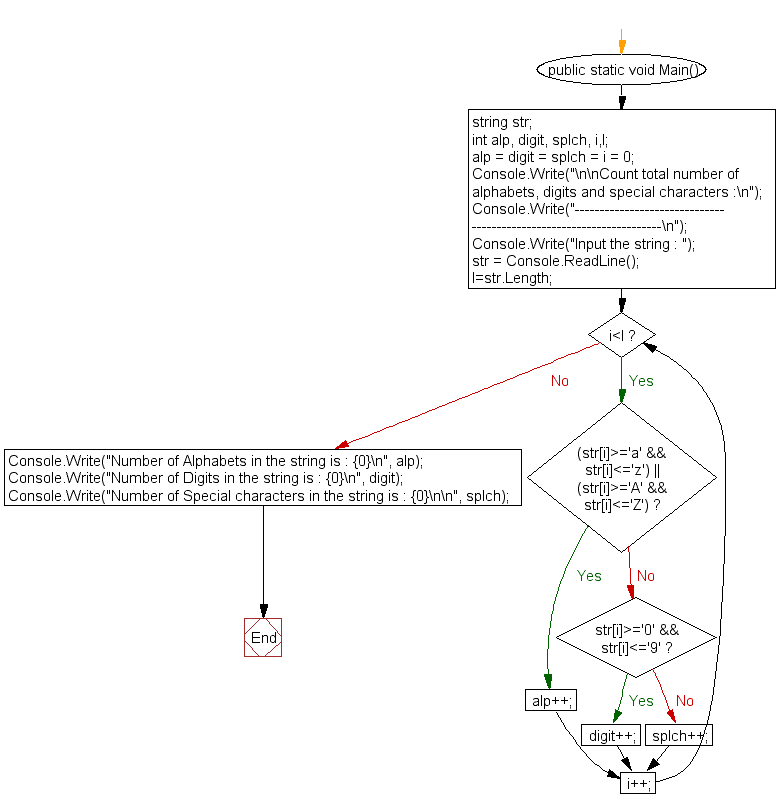
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to compare two string without using string library functions.
Next: Write a program in C# Sharp to copy one string to another string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.