C# Sharp Exercises: Check if a string has all unique characters
Write a C# Sharp program to check whether all characters in a given string are unique. If all the characters are distinct, return “There is no similarity between any of the characters!” otherwise if not unique, then:
- Display a message stating this.
- Display the duplicated character.
- It is only necessary to display the first non-unique character.
- Display where "both" duplicated characters appears in the string.
- All of the above messages can be incorporated into a single message.
Sample Solution:
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Define an array of strings containing various test cases
string[] text = { "", ".", "cppCPP", "PQR RQP", "The quick brown fox jumps over the lazy dog.", "C Sharp" };
// Iterate through each string in the 'text' array
foreach (string word in text)
{
// Display the current string, its length, and the characters with their positions having similarities
Console.WriteLine($"\n\"{word}\" (Length {word.Length}) " +
string.Join(", ",
// Select each character along with its index in the string
word.Select((ch, i) => new { ch, i })
// Group characters by their value and filter groups with more than one occurrence
.GroupBy(tm => tm.ch).Where(gr => gr.Count() > 1)
// Select groups and construct strings showing characters with their positions
.Select(gr => $"'{gr.Key}'[{string.Join(", ", gr.Select(tm => tm.i))}]")
// If no similarity between characters found, display a default message
.DefaultIfEmpty("There is no similarity between any of the characters!")));
}
}
}
}
Sample Output:
"" (Length 0) There is no similarity between any of the characters! "." (Length 1) There is no similarity between any of the characters! "cppCPP" (Length 6) 'p'[1, 2], 'P'[4, 5] "PQR RQP" (Length 7) 'P'[0, 6], 'Q'[1, 5], 'R'[2, 4] "The quick brown fox jumps over the lazy dog." (Length 44) 'h'[1, 32], 'e'[2, 28, 33], ' '[3, 9, 15, 19, 25, 30, 34, 39], 'u'[5, 21], 'r'[11, 29], 'o'[12, 17, 26, 41] "C Sharp" (Length 7) There is no similarity between any of the characters!
Flowchart :
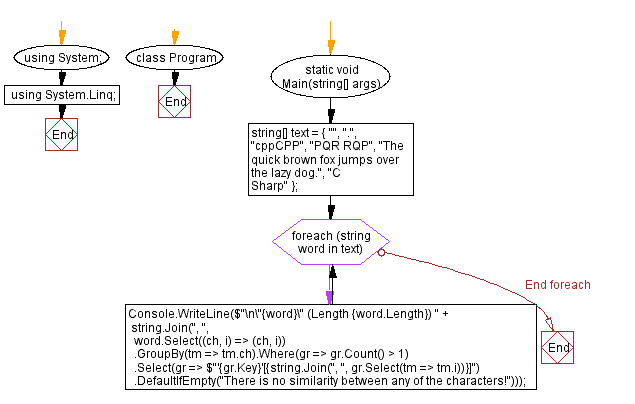
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous C# Sharp Exercise: Convert ASCII text to hexadecimal value as a string.
Next C# Sharp Exercise: C# Sharp programming exercises Function with Solution.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics