C#: Number of times a substring appeared in a string
Write a C# Sharp program that takes a string that repeats a substring. Count the number of times the substring appears.
Sample Data:
("aaaaaa") -> 6
("abababab") -> 4
("abcdabcdabcd") -> 3
Sample Solution-1:
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Declare and initialize a string variable
string text = "aaaaaa";
// Display the original string
Console.WriteLine("Original strings: " + text);
// Display the number of times a substring appeared in the string
Console.WriteLine("Number of times a substring appeared in the said string: " + test(text));
// Change the value of text
text = "abababab";
// Display the new original string
Console.WriteLine("Original strings: " + text);
// Display the number of times a substring appeared in the string
Console.WriteLine("Number of times a substring appeared in the said string: " + test(text));
// Change the value of text
text = "abcdabcdabcd";
// Display the new original string
Console.WriteLine("Original strings: " + text);
// Display the number of times a substring appeared in the string
Console.WriteLine("Number of times a substring appeared in the said string: " + test(text));
}
// Define a method 'test' that takes a string argument 'text' and returns an integer
public static int test(string text)
{
// Split the string by its first character, then count the number of resulting substrings
// The number of times a substring appeared in the string is equal to the count of substrings minus 1
return text.Split(text[0]).Count() - 1;
}
}
}
Sample Output:
Original strings: aaaaaa Number of times a substring appeared in the said string: 6 Original strings: abababab Number of times a substring appeared in the said string: 4 Original strings: abcdabcdabcd Number of times a substring appeared in the said string: 3
Flowchart :
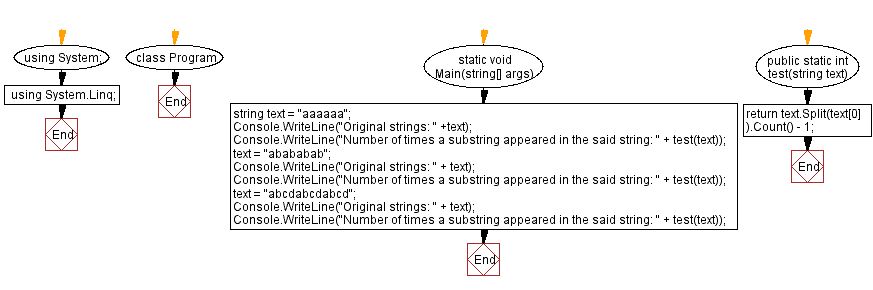
Sample Solution-2:
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Declare and initialize a string variable
string text = "aaaaaa";
// Display the original string
Console.WriteLine("Original strings: " + text);
// Display the number of times a substring appeared in the string
Console.WriteLine("Number of times a substring appeared in the said string: " + test(text));
// Change the value of text
text = "abababab";
// Display the new original string
Console.WriteLine("Original strings: " + text);
// Display the number of times a substring appeared in the string
Console.WriteLine("Number of times a substring appeared in the said string: " + test(text));
// Change the value of text
text = "abcdabcdabcd";
// Display the new original string
Console.WriteLine("Original strings: " + text);
// Display the number of times a substring appeared in the string
Console.WriteLine("Number of times a substring appeared in the said string: " + test(text));
}
// Define a method 'test' that takes a string argument 'text' and returns an integer
public static int test(string text)
{
// Find the index of the first character of the string starting from index 1 (excluding the first character)
int ctr = text.IndexOf(text[0], 1);
// If the index is less than 0, return 1 (as there's only one repeated substring)
// Otherwise, return the length of the string divided by the index of the second occurrence
return ctr < 0 ? 1 : text.Length / ctr;
}
}
}
Sample Output:
Original strings: aaaaaa Number of times a substring appeared in the said string: 6 Original strings: abababab Number of times a substring appeared in the said string: 4 Original strings: abcdabcdabcd Number of times a substring appeared in the said string: 3
Flowchart :
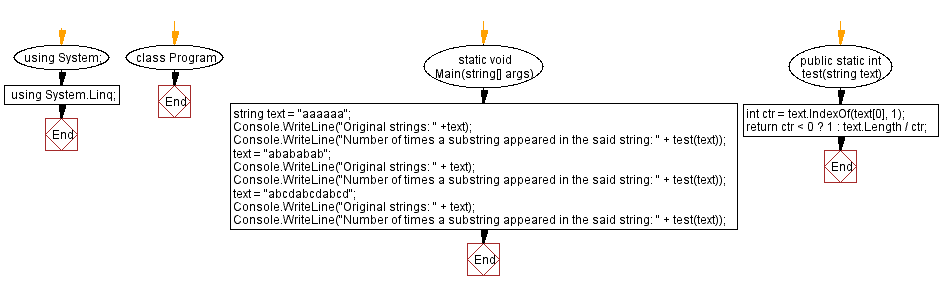
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous C# Sharp Exercise: Alphabet position in a string.
Next C# Sharp Exercise: Longest abecedarian word in a array of words.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics