C#: Count Occurrences of a substring in a string
Write a C# Sharp program to count a specific string (case sensitive) in given text.
Sample Solution-1:
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Declare and initialize string variables
string text = "The quick brown fox jumps over the lazy dog.";
string search_str = "The";
// Display the original string and search string
Console.WriteLine("Original string and search string:\n" + text + " \n" + search_str);
// Display the occurrences of the search string in the original string
Console.WriteLine("Occurrences of the said substring in the string: " + test(text, search_str));
// Change the values of text and search_str
text = "Red Green Black RedRed";
search_str = "Red";
// Display the new original string and search string
Console.WriteLine("\nOriginal string and search string:\n" + text + " \n" + search_str);
// Display the occurrences of the search string in the original string
Console.WriteLine("Occurrences of the said substring in the string: " + test(text, search_str));
// Change the values of text and search_str
text = "Red Green Black";
search_str = "Pink";
// Display the new original string and search string
Console.WriteLine("\nOriginal string and search string:\n" + text + " \n" + search_str);
// Display the occurrences of the search string in the original string
Console.WriteLine("Occurrences of the said substring in the string: " + test(text, search_str));
}
// Define a method 'test' that takes two string arguments and returns an integer
public static int test(string text, string search_str)
{
int count = 0;
// Loop through the characters of the original string
for (int i = 0; i < text.Length - search_str.Length + 1; i++)
{
// Check if the substring from the current position matches the search string
if (text.Substring(i, search_str.Length) == search_str)
{
count++; // Increment the count if a match is found
}
}
return count; // Return the total count of occurrences
}
}
}
Sample Output:
Original string and search string: The quick brown fox jumps over the lazy dog. The Occurrences of the said substring in the string: 1 Original string and search string: Red Green Black RedRed Red Occurrences of the said substring in the string: 3 Original string and search string: Red Green Black Pink Occurrences of the said substring in the string: 0
Flowchart :
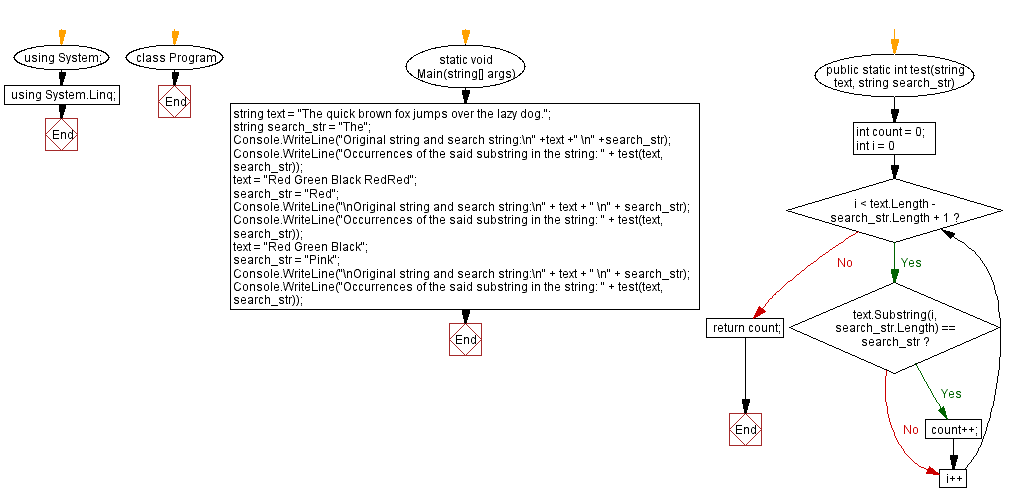
Sample Solution-2:
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Declare and initialize string variables
string text = "The quick brown fox jumps over the lazy dog.";
string search_str = "The";
// Display the original string and search string
Console.WriteLine("Original string and search string:\n" + text + " \n" + search_str);
// Display the occurrences of the search string in the original string
Console.WriteLine("Occurrences of the said substring in the string: " + test(text, search_str));
// Change the values of text and search_str
text = "Red Green Black RedRed";
search_str = "Red";
// Display the new original string and search string
Console.WriteLine("\nOriginal string and search string:\n" + text + " \n" + search_str);
// Display the occurrences of the search string in the original string
Console.WriteLine("Occurrences of the said substring in the string: " + test(text, search_str));
// Change the values of text and search_str
text = "Red Green Black";
search_str = "Pink";
// Display the new original string and search string
Console.WriteLine("\nOriginal string and search string:\n" + text + " \n" + search_str);
// Display the occurrences of the search string in the original string
Console.WriteLine("Occurrences of the said substring in the string: " + test(text, search_str));
}
// Define a method 'test' that takes two string arguments and returns an integer
public static int test(string text, string search_str)
{
// Calculate occurrences by finding the difference in lengths between the original string and a modified string
// The modified string is created by removing all occurrences of the search string and dividing by its length
return (text.Length - text.Replace(search_str, "").Length) / search_str.Length;
}
}
}
Sample Output:
Original string and search string: The quick brown fox jumps over the lazy dog. The Occurrences of the said substring in the string: 1 Original string and search string: Red Green Black RedRed Red Occurrences of the said substring in the string: 3 Original string and search string: Red Green Black Pink Occurrences of the said substring in the string: 0
Flowchart :
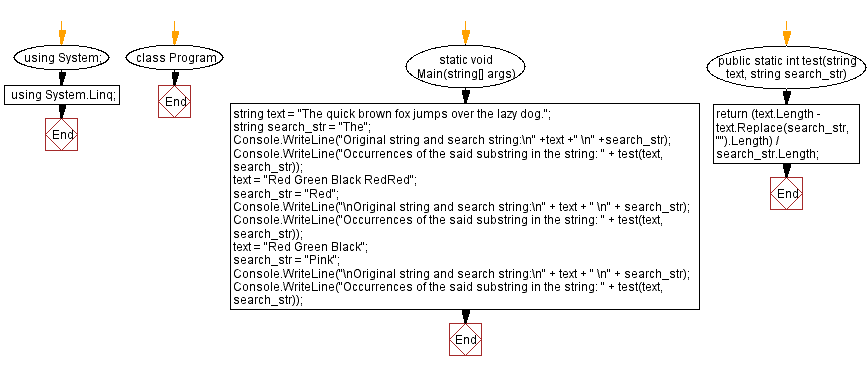
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous C# Sharp Exercise: Count the number of duplicate characters in a string.
Next C# Sharp Exercise: Alphabet position in a string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics