C#: Count the number of duplicate characters in a string
Write a C# Sharp program to count the number of duplicate characters (case sensitive) including spaces in a given string. If there are no duplicates, return 0.
Sample Data:
("Red Green WHITE") -> 2
("ppqrrsttuuu") -> 4
("dow jones industrial average") -> 9
Sample Solution-1:
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Declare and initialize a string variable
string text = "Red Green WHITE";
// Display the original string
Console.WriteLine("Original String: " + text);
// Display the number of duplicate characters in the string
Console.WriteLine("Number of duplicate characters in the said string: " + test(text));
// Change the value of text
text = "ppqrrsttuuu";
// Display the new original string
Console.WriteLine("Original String: " + text);
// Display the number of duplicate characters in the string
Console.WriteLine("Number of duplicate characters in the said string: " + test(text));
// Change the value of text
text = "dow jones industrial average";
// Display the new original string
Console.WriteLine("Original String: " + text);
// Display the number of duplicate characters in the string
Console.WriteLine("Number of duplicate characters in the said string: " + test(text));
}
// Define a method 'test' that takes a string argument 'text' and returns an integer
public static int test(string text)
{
// Use LINQ to group characters in the string and count the number of groups with more than or equal to two occurrences
return text.GroupBy(n => n).Count(n => n.Count() >= 2);
}
}
}
Sample Output:
Original String: Red Green WHITE Number of duplicate characters in the said string: 2 Original String: ppqrrsttuuu Number of duplicate characters in the said string: 4 Original String: dow jones industrial average Number of duplicate characters in the said string: 9
Flowchart :
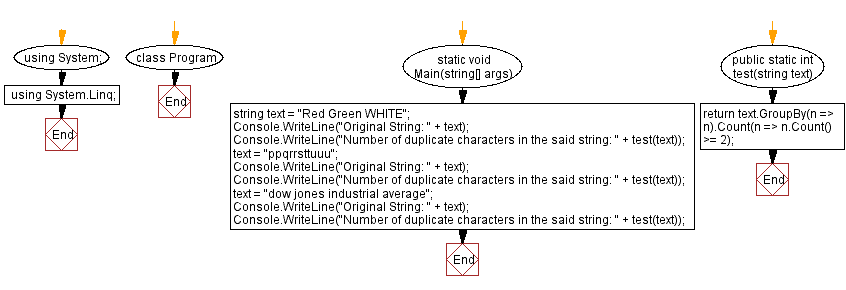
Sample Solution-2:
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Declare and initialize a string variable
string text = "Red Green WHITE";
// Display the original string
Console.WriteLine("Original String: " + text);
// Display the number of duplicate characters in the string
Console.WriteLine("Number of duplicate characters in the said string: " + test(text));
// Change the value of text
text = "ppqrrsttuuu";
// Display the new original string
Console.WriteLine("Original String: " + text);
// Display the number of duplicate characters in the string
Console.WriteLine("Number of duplicate characters in the said string: " + test(text));
// Change the value of text
text = "dow jones industrial average";
// Display the new original string
Console.WriteLine("Original String: " + text);
// Display the number of duplicate characters in the string
Console.WriteLine("Number of duplicate characters in the said string: " + test(text));
}
// Define a method 'test' that takes a string argument 'text' and returns an integer
public static int test(string text)
{
// Using LINQ, group characters in the string and filter groups with more than one occurrence
var t = from x in text
group x by x into gr
where gr.Count() > 1
select gr;
// Return the count of such groups (representing duplicate characters)
return (t.Count());
}
}
}
Sample Output:
Original String: Red Green WHITE Number of duplicate characters in the said string: 2 Original String: ppqrrsttuuu Number of duplicate characters in the said string: 4 Original String: dow jones industrial average Number of duplicate characters in the said string: 9
Flowchart :
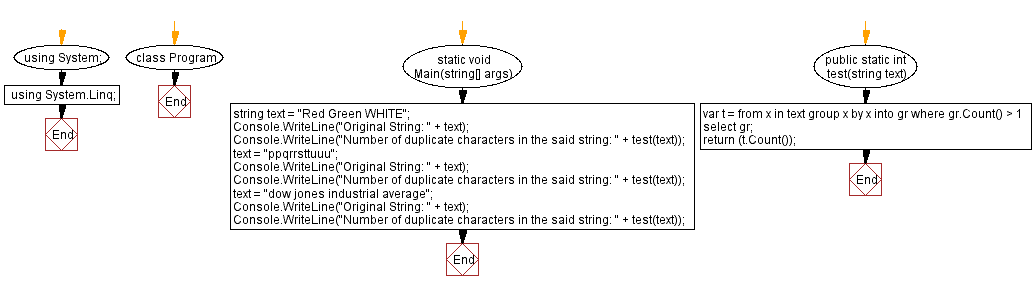
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous C# Sharp Exercise: Reverse an integer and add with the original number.
Next C# Sharp Exercise: Count Occurrences of a substring in a string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics