C#: Compare two string whether they are equal or not
Write a program in C# Sharp to compare two strings without using a string library functions.
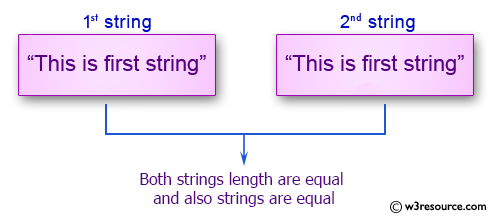
Sample Solution:-
C# Sharp Code:
using System;
// Define the Exercise6 class
public class Exercise6
{
// Main method - entry point of the program
public static void Main()
{
string str1, str2; // Declare two string variables
int flg = 0; // Initialize a flag variable to determine the comparison result
int i = 0, l1, l2, yn = 0; // Declare variables for iteration and string lengths
// Prompt the user to compare two strings
Console.Write("\n\nCompare two strings whether they are equal or not:\n");
Console.Write("------------------------------------------------------\n");
Console.Write("Input the 1st string: ");
str1 = Console.ReadLine(); // Read the first input string
Console.Write("Input the 2nd string: ");
str2 = Console.ReadLine(); // Read the second input string
l1 = str1.Length; // Get the length of the first string
l2 = str2.Length; // Get the length of the second string
// Compare checking when the strings are equal in length
if (l1 == l2)
{
for (i = 0; i < l1; i++)
{
if (str1[i] != str2[i])
{
yn = 1;
i = l1; // Exit the loop if a difference in characters is found
}
}
}
// Initialize the flag where they are equal, smaller, or greater in length
if (l1 == l2)
flg = 0;
else if (l1 > l2)
flg = 1;
else if (l1 < l2)
flg = -1;
// Display messages based on the comparison result
if (flg == 0)
{
if (yn == 0)
Console.Write("\nThe length of both strings are equal and both strings are same.\n\n");
else
Console.Write("\nThe length of both strings are equal but they are not same.\n\n");
}
else if (flg == -1)
{
Console.Write("\nThe length of the first string is smaller than the second.\n\n");
}
else
{
Console.Write("\nThe length of the first string is greater than the second.\n\n");
}
}
}
Sample Output:
Compare two strings whether they are equal or not : ------------------------------------------------------ Input the 1st string : This is the first string. Input the 2nd string : This is the second string. The length of the first string is smaller than second.
Flowchart:
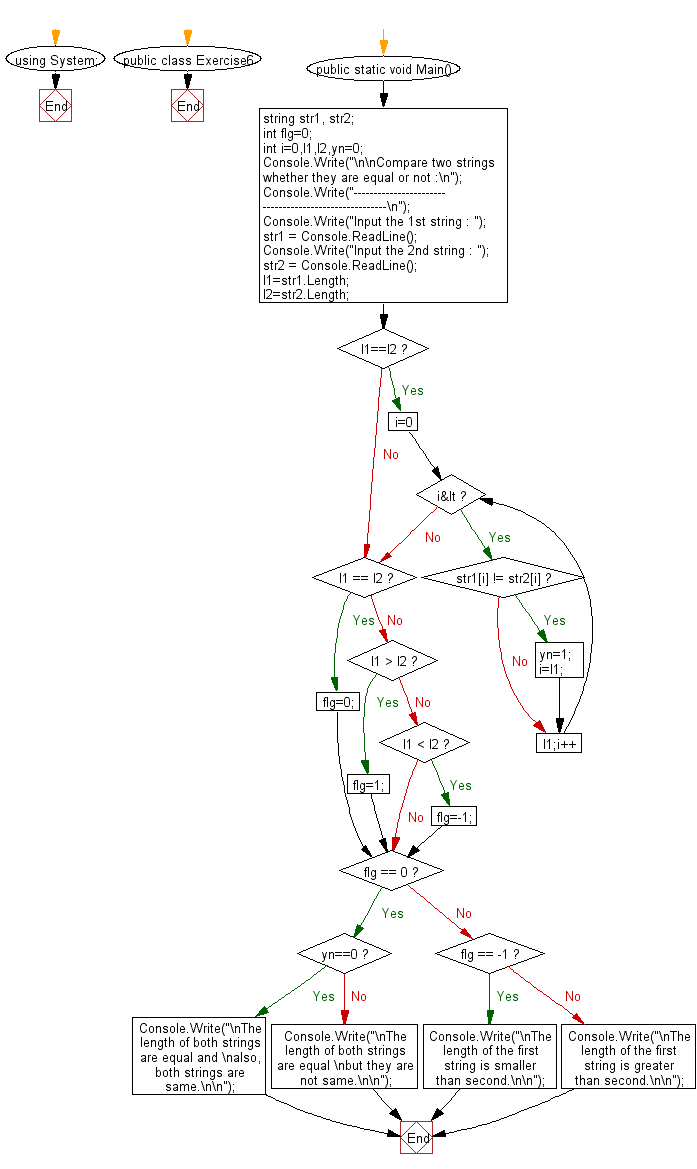
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to count the total number of words in a string.
Next: Write a program in C# Sharp to count a total number of alphabets, digits and special characters in a string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics