C#: Check if a string is an anagram of another given string
From Wikipedia-
An anagram is a word or phrase formed by rearranging the letters of a different word or phrase, typically using all the original letters exactly once. For example, the word anagram itself can be rearranged into nag a ram, also the word binary into brainy and the word adobe into abode.
Write a C# Sharp program to check if two given strings are anagrams or not.
Sample Data:
("wxyz", "zyxw") -> True
("pears", "spare") -> True
("stone", "tones") -> True
("cat", "rat") -> False
Sample Solution:
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Declare and initialize two string variables
string text1 = "wxyz";
string text2 = "zyxw";
// Display the original strings
Console.WriteLine("Original strings: " + text1 + " " + text2);
// Check if the two strings are anagrams and display the result
Console.WriteLine("Check if two said strings are anagrams or not: " + test(text1, text2));
// Change the values of text1 and text2
text1 = "pears";
text2 = "spare";
// Display the new original strings
Console.WriteLine("\nOriginal strings: " + text1 + " " + text2);
// Check if the two strings are anagrams and display the result
Console.WriteLine("Check if two said strings are anagrams or not: " + test(text1, text2));
// Change the values of text1 and text2
text1 = "stone";
text2 = "tones";
// Display the new original strings
Console.WriteLine("\nOriginal strings: " + text1 + " " + text2);
// Check if the two strings are anagrams and display the result
Console.WriteLine("Check if two said strings are anagrams or not: " + test(text1, text2));
// Change the values of text1 and text2
text1 = "cat";
text2 = "rat";
// Display the new original strings
Console.WriteLine("\nOriginal strings: " + text1 + " " + text2);
// Check if the two strings are anagrams and display the result
Console.WriteLine("Check if two said strings are anagrams or not: " + test(text1, text2));
}
// Define a method 'test' that takes two string arguments and returns a boolean indicating if they are anagrams
public static bool test(string text1, string text2)
{
// Convert both strings to uppercase, sort the characters, and concatenate them
string stext1 = String.Concat(text1.ToUpper().OrderBy(c => c));
string stext2 = String.Concat(text2.ToUpper().OrderBy(c => c));
// Compare the sorted and concatenated strings, return true if they are equal (anagrams), otherwise return false
if (stext1 == stext2)
{
return true;
}
else
{
return false;
}
}
}
}
Sample Output:
Original strings: wxyz zyxw Check if two said strings are anagrams or not: True Original strings: pears spare Check if two said strings are anagrams or not: True Original strings: stone tones Check if two said strings are anagrams or not: True Original strings: cat rat Check if two said strings are anagrams or not: False
Flowchart :
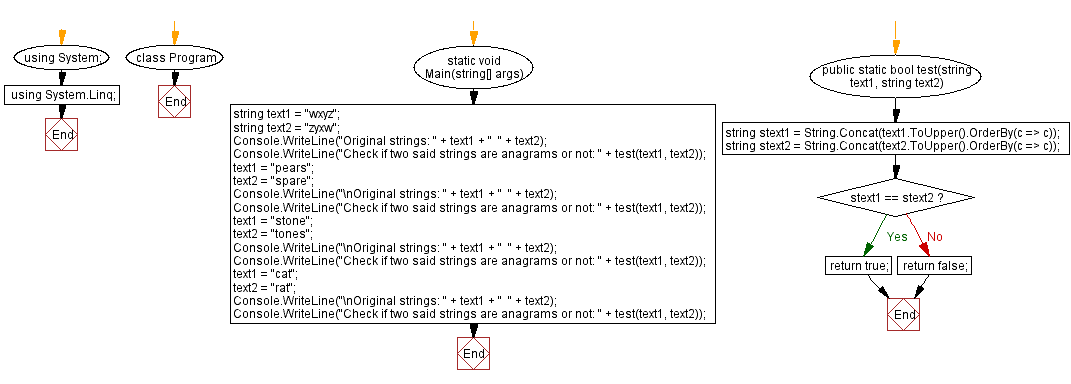
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Reverse the words of three or more lengths in a string.
Next: Reverse an integer and add with the original number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.