C#: Find the position of a specified word in a given string
Write a C# Sharp program to find the position of a specified word in a given string.
Sample Example: | |||||||||
Text: | The | quick | brown | fox | jumps | over | the | lazy | dog. |
Position: | 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 |
Sample Solution:-
C# Sharp Code:
using System;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Declare and initialize a string variable
string str1 = "The quick brown fox jumps over the lazy dog.";
// Display the original string
Console.WriteLine("Original string: " + str1);
// Display the position of the word 'fox' in the string
Console.WriteLine("Position of the word 'fox' in the said string: " + test(str1, "fox"));
// Display the position of the word 'The' in the string
Console.WriteLine("Position of the word 'The' in the said string: " + test(str1, "The"));
// Display the position of the word 'lazy' in the string
Console.WriteLine("Position of the word 'lazy' in the said string: " + test(str1, "lazy"));
}
// Define a method 'test' that takes a string argument 'text' and a string argument 'word', and returns the position of the word in the text
public static int test(string text, string word)
{
// Split the input text into words and find the index (position) of the specified word
// Adding +1 to make the position index human-readable (starting from 1 instead of 0)
return Array.IndexOf(text.Split(' '), word) + 1;
}
}
}
Sample Output:
Original string: The quick brown fox jumps over the lazy dog. Position of the word 'fox' in the said string: 4 Position of the word 'The' in the said string: 1 Position of the word 'lazy' in the said string: 8
Flowchart :
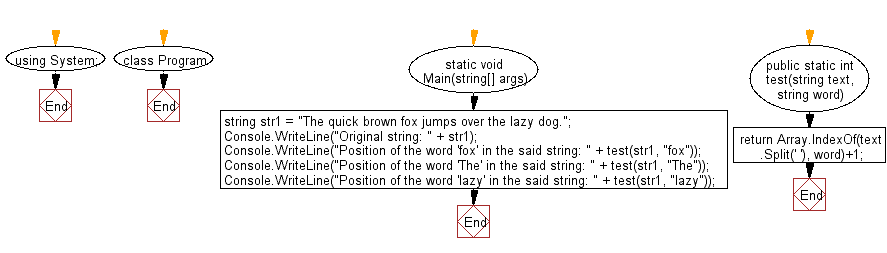
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to convert the first character of each word of a given string to uppercase.
Next: Write a C# Sharp program to alternate the case of each letter in a given string and the first letter of the said string must be uppercase.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.