C#: Print individual characters of the string in reverse order
Write a program in C# Sharp to print individual characters of the string in reverse order.
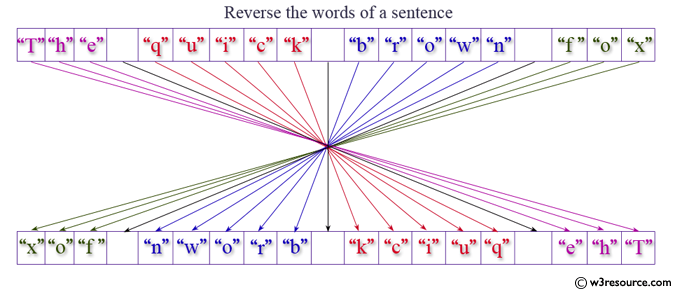
Sample Solution:-
C# Sharp Code:
using System;
// Define the Exercise4 class
public class Exercise4
{
// Main method - entry point of the program
public static void Main()
{
string str; // Declare a string variable
int l = 0; // Initialize a variable to traverse the string characters
// Prompt the user to print individual characters of the string in reverse order
Console.Write("\n\nPrint individual characters of the string in reverse order:\n");
Console.Write("------------------------------------------------------\n");
// Request user input for a string
Console.Write("Input the string: ");
str = Console.ReadLine(); // Read the user input
l = str.Length - 1; // Get the last index of the string
Console.Write("The characters of the string in reverse are:\n");
// Loop through the string in reverse to print individual characters
while (l >= 0)
{
Console.Write("{0} ", str[l]); // Display each character in reverse order with a space
l--; // Move to the previous character in the string
}
Console.Write("\n\n"); // Add newline for formatting
}
}
Sample Output:
print individual characters of string in reverse order : ------------------------------------------------------ Input the string : ecruoser3w The characters of the string in reverse are : w 3 r e s o u r c e
Flowchart:
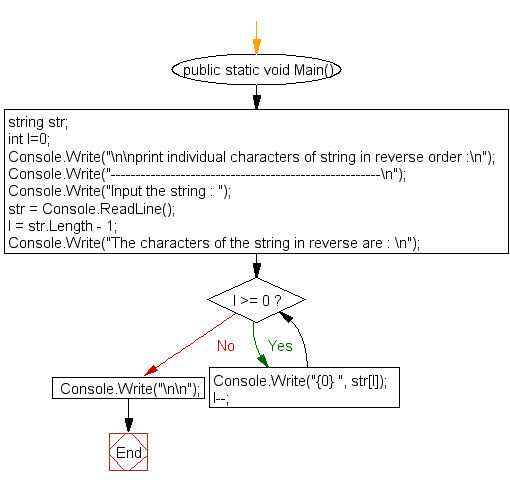
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to separate the individual characters from a string.
Next: Write a program in C# Sharp to count the total number of words in a string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.