C#: Demonstrate how culture can affect a comparison
C# Sharp String: Exercise-27 with Solution
Write a C# Sharp program to demonstrate how culture affects comparisons.
Note : In Czech – Czech Republic culture, "ch" is a single character that is greater than "d". However, in English - United States culture, "ch" consists of two characters, and "c" is less than "d".
Sample Solution:-
C# Sharp Code:
using System;
using System.Globalization;
class Example27
{
public static void Main() {
// Declare and initialize two strings for comparison
String str1 = "change";
String str2 = "dollar";
// Declare a variable to hold the relationship between the strings
String relation = null;
// Perform string comparison for "en-US" culture
relation = symbol(String.Compare(str1, str2, false, new CultureInfo("en-US")));
// Display the relationship between str1 and str2 for the "en-US" culture
Console.WriteLine("\nFor en-US: {0} {1} {2}", str1, relation, str2);
// Perform string comparison for "cs-CZ" culture
relation = symbol(String.Compare(str1, str2, false, new CultureInfo("cs-CZ")));
// Display the relationship between str1 and str2 for the "cs-CZ" culture
Console.WriteLine("For cs-CZ: {0} {1} {2}\n", str1, relation, str2);
}
// Method to convert comparison result to symbols (<, =, >)
private static String symbol(int r) {
String s = "=";
if (r < 0)
s = "<"; // If result is less than 0, set symbol to <
else if (r > 0)
s = ">"; // If result is greater than 0, set symbol to >
return s; // Return the symbol
}
}
Sample Output:
For en-US: change < dollar For cs-CZ: change > dollar
Flowchart :
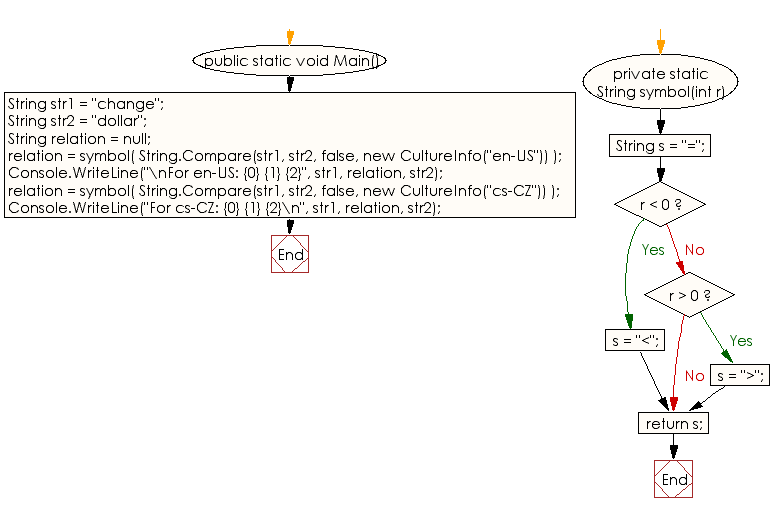
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write C# Sharp program to demonstrate that the Compare(String, String, Boolean) method is equivalent to using ToUpper or ToLower when comparing strings.
Next: Write a C# Sharp program to compare two strings in following three different ways produce three different results.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/csharp-exercises/string/csharp-string-exercise-27.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics