C#: Compare the last names and lists them in alphabetical order
Write a C# Sharp program to compare the last names of two people. It then lists them in alphabetical order.
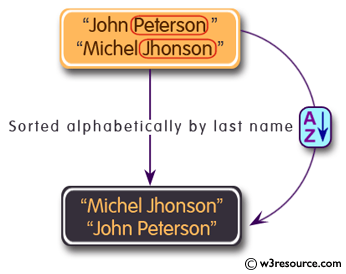
Sample Solution:-
C# Sharp Code:
using System;
using System.Globalization;
public class Example24
{
public static void Main()
{
// Define and initialize two name strings
string name1 = "John Peterson";
string name2 = "Michel Jhonson";
// Get position of space character in name1.
int index1 = name1.IndexOf(" ");
index1 = index1 < 0 ? 0 : index1--; // Decrement the index1 value (unless it's negative)
// Get position of space character in name2.
int index2 = name2.IndexOf(" ");
index2 = index2 < 0 ? 0 : index2--; // Decrement the index2 value (unless it's negative)
// Determine the maximum length between name1 and name2
int length = Math.Max(name1.Length, name2.Length);
// Display the names sorted alphabetically by last name
Console.WriteLine("Sorted alphabetically by last name:");
// Compare the last names of name1 and name2 using the specified culture and ignore case
if (String.Compare(name1, index1, name2, index2, length, new CultureInfo("en-US"), CompareOptions.IgnoreCase) < 0)
Console.WriteLine("{0}\n{1}", name1, name2); // Display name1 first if it comes before name2
else
Console.WriteLine("{0}\n{1}", name2, name1); // Otherwise, display name2 first
}
}
Sample Output:
Sorted alphabetically by last name: Michel Jhonson John Peterson
Flowchart :
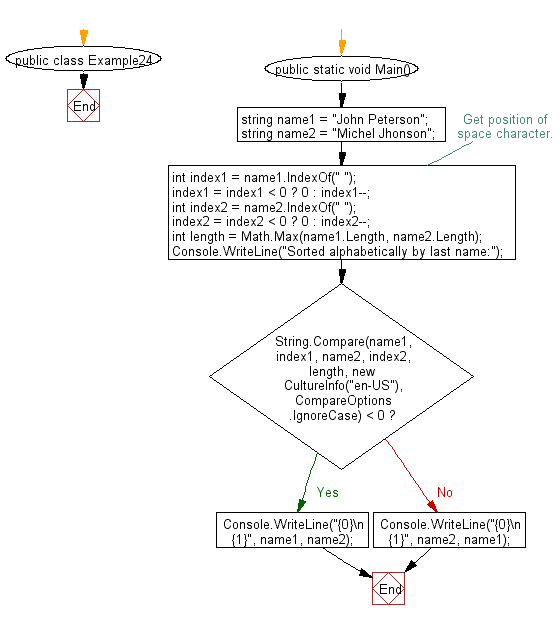
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to compare two substrings using different cultures and ignoring the case of the substrings.
Next: Write a program in C# Sharp to insert a substring before the first occurence of a string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.