C#: Find the length of a string
Write a C# Sharp program to find the length of a string without using a library function.
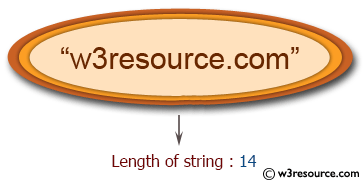
Sample Solution:-
C# Sharp Code:
using System;
// Define the Exercise2 class
public class Exercise2
{
// Main method - entry point of the program
public static void Main()
{
string str; // Declare a string variable
int l = 0; // Initialize a variable to store the length of the string
// Prompt the user to find the length of a string
Console.Write("\n\nFind the length of a string:\n");
Console.Write("---------------------------------\n");
// Request user input for a string
Console.Write("Input the string: ");
str = Console.ReadLine(); // Read the user input
// Loop through each character in the string to calculate its length
foreach (char chr in str)
{
l += 1; // Increment the length counter for each character encountered
}
// Display the length of the entered string
Console.Write("Length of the string is: {0}\n\n", l);
}
}
Sample Output:
Find the length of a string : --------------------------------- Input the string : w3resource Length of the string is : 10
Flowchart:
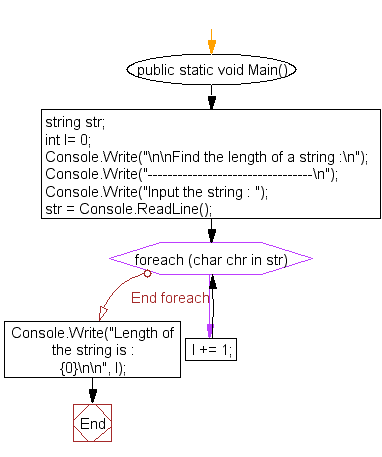
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to input a string and print it.
Next: Write a program in C# Sharp to separate the individual characters from a string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics