C#: Check whether a character is an alphabet or not and if so, check for the case
Write a C# Sharp program to check whether a character is an alphabet and not and if so, check for the case.
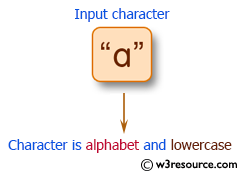
Sample Solution:-
C# Sharp Code:
using System;
// Define the Exercise18 class
public class Exercise18
{
// Main method - entry point of the program
static void Main()
{
// Prompt the user to check whether a character is an alphabet or not
Console.Write("\n\nCheck whether a character is alphabet or not and if so, check for case :\n");
Console.Write("-----------------------------------------------------------------------------\n");
// Prompt the user to input a character
Console.Write("Input a character: ");
// Read a single character from the console input
char ch = (char)Console.Read();
// Check if the entered character is an alphabet
if (Char.IsLetter(ch))
{
// Check if the alphabet is uppercase
if (Char.IsUpper(ch))
{
// Display a message indicating the character is uppercase
Console.WriteLine("\nThe character is uppercase.\n");
}
else
{
// Display a message indicating the character is lowercase
Console.WriteLine("\nThe character is lowercase.\n");
}
}
else
{
// Display a message indicating that the entered character is not an alphabet
Console.WriteLine("\nThe entered character is not an alphabetic character.\n");
}
}
}
Sample Output:
Check whether a character is alphabet or not and if so, check for case : ----------------------------------------------------------------------------- Input a character: a The character is lowercase.
Flowchart:
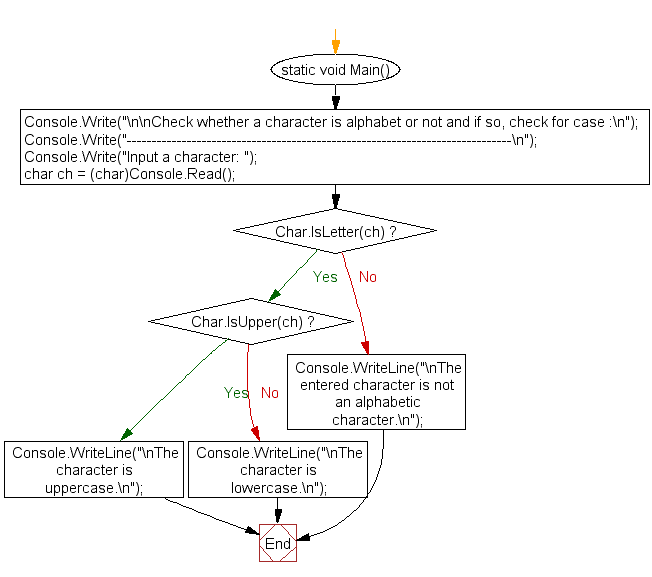
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to search the position of a substring within a string.
Next: Write a program in C# Sharp to find the number of times a substring appears in the given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.