C#: Find the maximum element in a stack
Write a C# program to find the maximum element in a stack.
Sample Solution:
C# Code:
using System;
// Implementation of a Stack data structure
public class Stack
{
private int[] items; // Array to hold stack elements
private int top; // Index representing the top of the stack
// Constructor to initialize the stack with a specified size
public Stack(int size)
{
items = new int[size]; // Initializing the array with the given size
top = -1; // Initializing top to -1, indicating an empty stack
}
// Method to check if the stack is empty
public bool IsEmpty()
{
return top == -1; // Returns true if top is -1 (empty stack), otherwise false
}
// Method to check if the stack is full
public bool IsFull()
{
return top == items.Length - 1; // Returns true if top is at the last index of the array (full stack)
}
// Method to push an element onto the stack
public void Push(int item)
{
if (IsFull())
{
Console.WriteLine("Stack Full!"); // Displays a message if the stack is full
return;
}
items[++top] = item; // Inserts the item at the incremented top index
}
// Method to pop an element from the stack
public int Pop()
{
if (IsEmpty())
{
Console.WriteLine("Stack underflow"); // Displays a message if the stack is empty
return -1;
}
return items[top--]; // Removes and returns the top element by decrementing top
}
// Method to peek at the top element of the stack without removing it
public int Peek()
{
if (IsEmpty())
{
Console.WriteLine("Stack is empty"); // Displays a message if the stack is empty
return -1;
}
return items[top]; // Returns the element at the top index without removing it
}
// Method to get the current size of the stack
public static int Size(Stack stack)
{
return stack.top + 1; // Returns the number of elements in the stack
}
// Method to find the maximum value in the stack
public static int Max(Stack stack)
{
if (stack.IsEmpty())
{
Console.WriteLine("Stack is empty"); // Displays a message if the stack is empty
return -1;
}
// Initialize max to the first element of the stack
int max = stack.Peek();
// Traverse the stack and compare each element to the current max
for (int i = stack.top; i >= 0; i--)
{
if (stack.items[i] > max)
{
max = stack.items[i]; // Update max if a larger element is found
}
}
return max; // Return the maximum element found in the stack
}
// Method to display the stack elements
public static void Display(Stack stack)
{
if (stack.IsEmpty())
{
Console.WriteLine("Stack is empty");
return;
}
Console.WriteLine("Stack elements:");
for (int i = stack.top; i >= 0; i--)
{
Console.Write(stack.items[i] + " "); // Displays each element in the stack
}
}
}
// Main class to demonstrate the functionality of the Stack class
public class Program
{
public static void Main(string[] args)
{
Console.WriteLine("Initialize a stack:");
Stack stack = new Stack(10); // Creating a stack with a size of 10
Console.WriteLine("Input some elements onto the stack:");
stack.Push(1);
stack.Push(3);
stack.Push(4);
stack.Push(9);
stack.Push(5);
stack.Push(6);
Stack.Display(stack); // Displaying the elements in the original stack
int max = Stack.Max(stack); // Finding the maximum element in the stack
Console.WriteLine("\nMaximum element in the stack: " + max);
}
}
Sample Output:
Initialize a stack: Input some elements onto the stack: Stack elements: 6 5 9 4 3 1 Maximum element in the stack: 9
Flowchart:
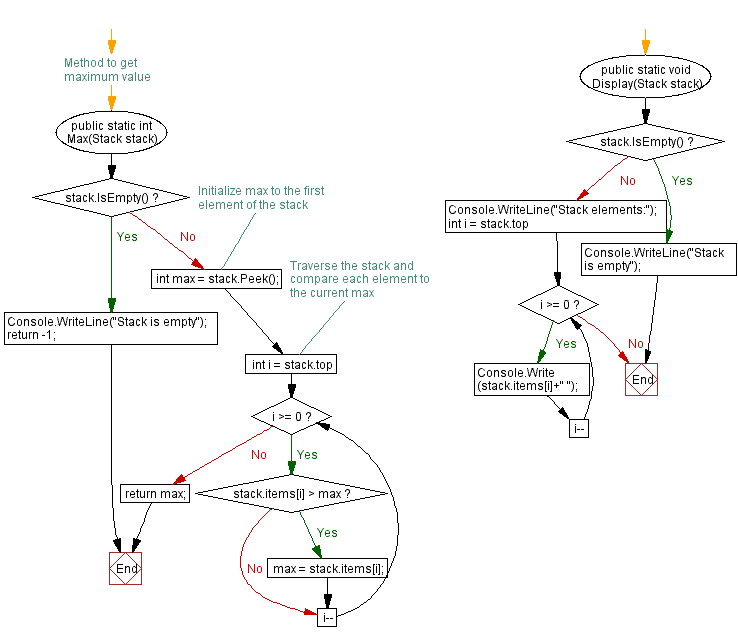
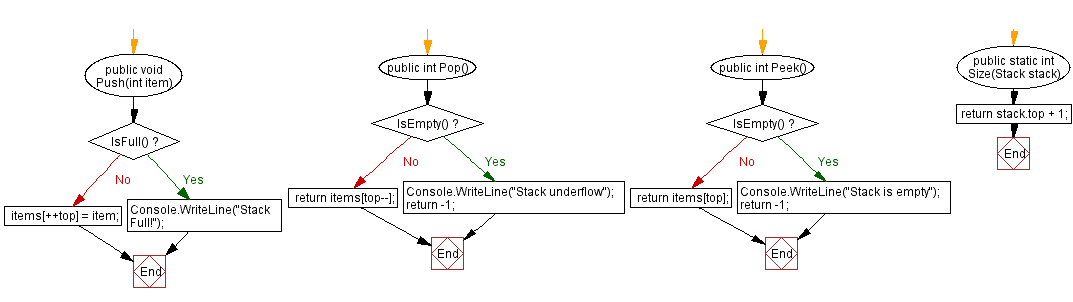
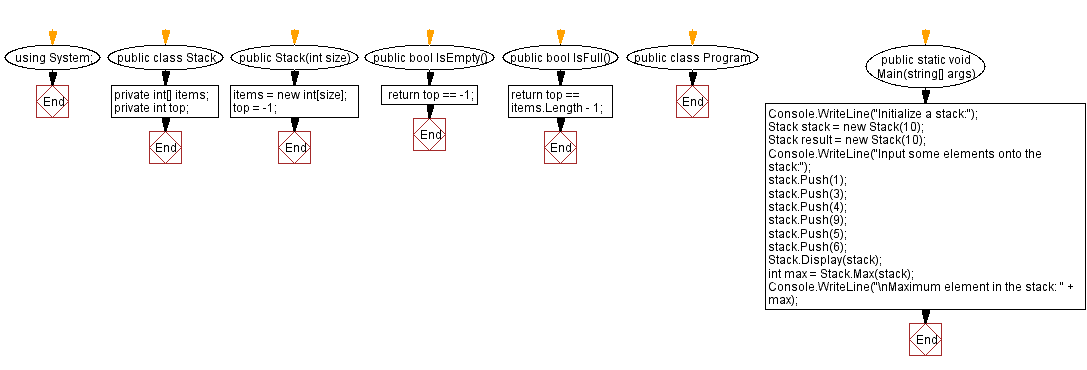
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Find the minimum element in a stack.
Next: Write a C# Sharp program to find the greater and smaller value of two variables.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.