C#: Verify all stack elements satisfy a condition
C# Sharp Stack: Exercise-25 with Solution
Write a C# program that implements a stack and checks if all elements of the stack satisfy a condition.
Sample Solution:
C# Code:
using System;
using System.Collections.Generic;
public class Stack
{
private int[] items; // Array to store stack elements
private int top; // Index indicating the top element in the stack
// Constructor to initialize the stack with a specified size
public Stack(int size)
{
items = new int[size]; // Create an array of integers with the given size
top = -1; // Initialize top index to -1, indicating an empty stack
}
// Check if the stack is empty
public bool IsEmpty()
{
return top == -1; // Returns true if the stack is empty
}
// Check if the stack is full
public bool IsFull()
{
return top == items.Length - 1; // Returns true if the stack is full
}
// Push an element onto the stack
public void Push(int item)
{
if (IsFull())
{
Console.WriteLine("Stack Full!"); // Display a message indicating stack overflow if the stack is full
return;
}
items[++top] = item; // Increment top and add the item to the stack
}
// Pop an element from the stack
public int Pop()
{
if (IsEmpty())
{
Console.WriteLine("Stack underflow"); // Display a message indicating stack underflow if the stack is empty
return -1;
}
return items[top--]; // Return and decrement top to remove the element from the stack
}
// Peek at the top element of the stack without removing it
public int Peek()
{
if (IsEmpty())
{
Console.WriteLine("Stack is empty"); // Display a message indicating the stack is empty
return -1;
}
return items[top]; // Return the top element of the stack
}
// Static method to get the size of a stack
public static int Size(Stack stack)
{
return stack.top + 1; // Return the size of the stack based on the current top index
}
// Method to count all the elements in a stack
public static int Count(Stack stack)
{
int count = 0;
Stack temp = new Stack(Size(stack));
// Move elements from the original stack to a temporary stack and count them
while (!stack.IsEmpty())
{
temp.Push(stack.Pop()); // Move elements from the original stack to the temporary stack
count++; // Increment count for each element moved
}
// Restore the original stack by moving elements back from the temporary stack
while (!temp.IsEmpty())
{
stack.Push(temp.Pop()); // Move elements back to the original stack
}
return count; // Return the total count of elements in the stack
}
// Method to verify if all stack elements satisfy a given condition
public static bool every(Stack stack, Func<int, bool> condition)
{
Stack temp = new Stack(Size(stack));
bool allSatisfy = true;
while (!stack.IsEmpty())
{
int item = stack.Pop();
if (!condition(item)) // Check if the condition is not satisfied for any element
{
allSatisfy = false; // Update flag to indicate not all elements satisfy the condition
}
temp.Push(item); // Push the element to the temporary stack
}
while (!temp.IsEmpty())
{
stack.Push(temp.Pop()); // Restore the original stack by moving elements back
}
return allSatisfy; // Return whether all elements satisfy the condition
}
// Method to display the elements of the stack
public static void Display(Stack stack)
{
if (stack.IsEmpty())
{
Console.WriteLine("Stack is empty"); // Display a message if the stack is empty
return;
}
Console.WriteLine("Stack elements:");
for (int i = stack.top; i >= 0; i--)
{
Console.Write(stack.items[i] + " "); // Display elements of the stack
}
}
}
public class Program
{
public static void Main(string[] args)
{
Console.WriteLine("Initialize three stacks:");
Stack stack1 = new Stack(10);
stack1.Push(1);
stack1.Push(2);
stack1.Push(3);
Console.Write("Stack1: ");
Stack.Display(stack1);
Stack stack2 = new Stack(10);
stack2.Push(2);
stack2.Push(3);
stack2.Push(4);
stack2.Push(5);
Console.Write("\nStack2: ");
Stack.Display(stack2);
Stack stack3 = new Stack(10);
stack3.Push(4);
stack3.Push(4);
stack3.Push(6);
Console.Write("\nStack3: ");
Stack.Display(stack3);
Console.WriteLine("\n\nVerify all stack elements are even:");
Console.Write("Stack1: ");
Console.WriteLine(Stack.every(stack1, x => x % 2 == 0));
Console.Write("Stack2: ");
Console.WriteLine(Stack.every(stack2, x => x % 2 == 0));
Console.Write("Stack3: ");
Console.WriteLine(Stack.every(stack3, x => x % 2 == 0)); ;
}
}
Sample Output:
Initialize three stacks: Stack1: Stack elements: 3 2 1 Stack2: Stack elements: 5 4 3 2 Stack3: Stack elements: 6 4 4 Verify all stack elements are even: Stack1: False Stack2: False Stack3: True
Flowchart:
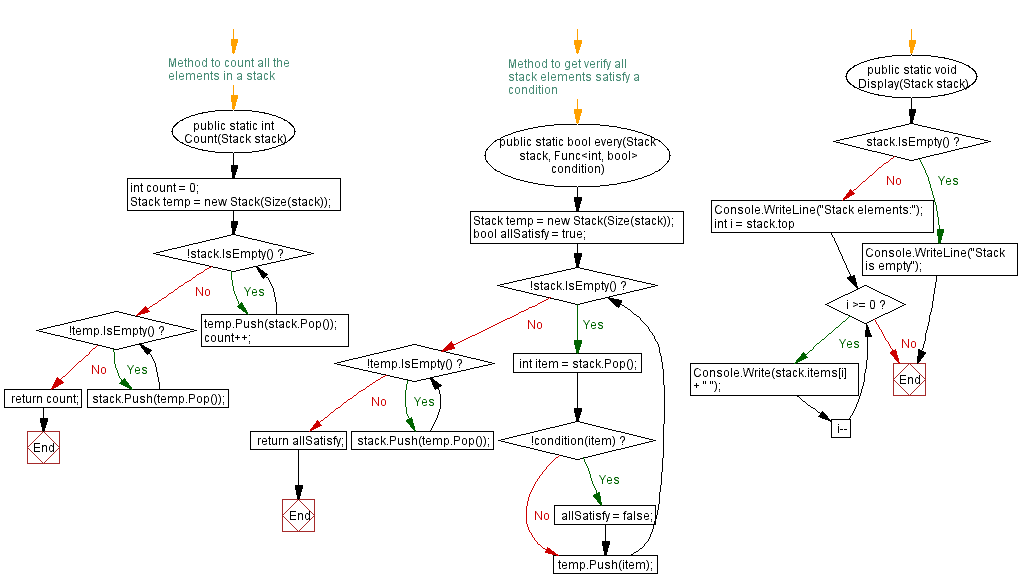
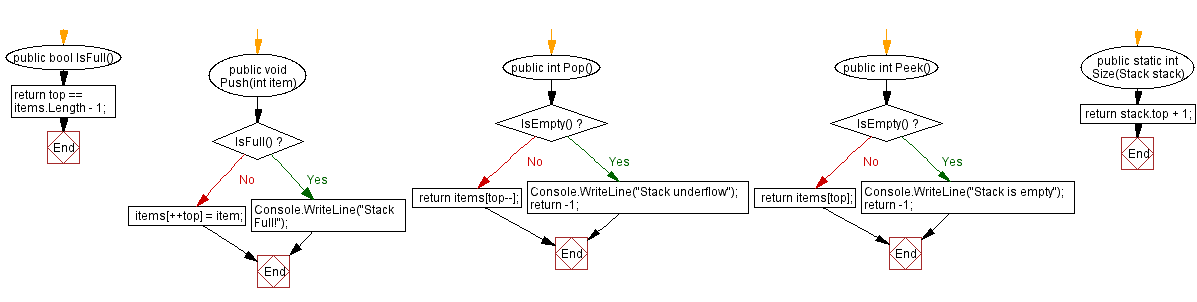
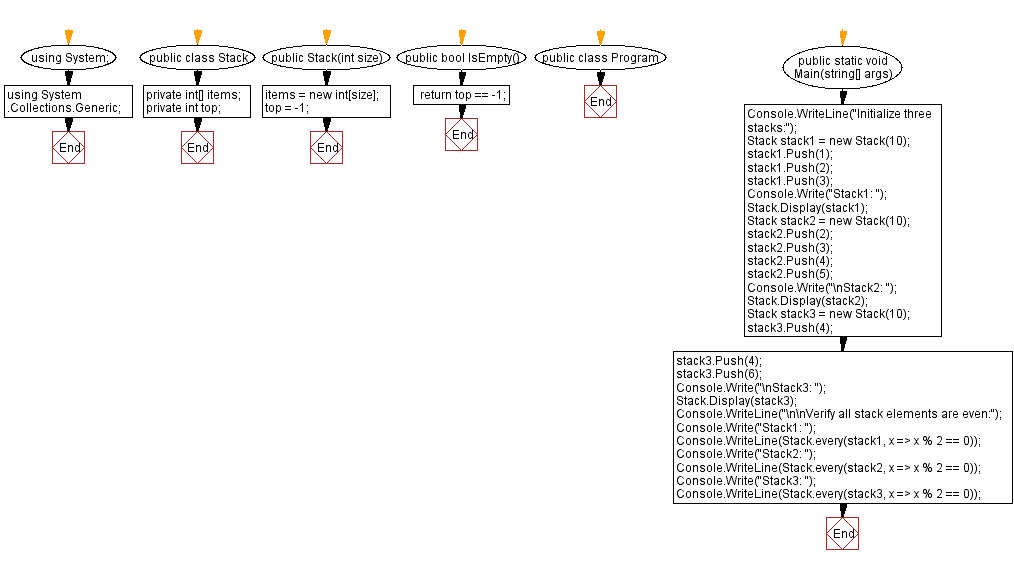
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: New stack from a portion of the original stack.
Next: Verify at least one element satisfy a condition.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/csharp-exercises/stack/csharp-stack-exercise-25.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics