C#: Elements from both stacks without duplicates
Write a C# program that implements a stack and creates a new stack that contains all elements from both stacks without duplicates.
Sample Solution:
C# Code:
using System;
using System.Collections.Generic;
public class Stack
{
private int[] items; // Array to store stack elements
private int top; // Index pointing to the top of the stack
// Constructor to initialize the stack with a specified size
public Stack(int size)
{
items = new int[size]; // Creating an array of integers with the given size
top = -1; // Initializing top index to -1 indicating an empty stack
}
// Check if the stack is empty
public bool IsEmpty()
{
return top == -1; // Returns true if the stack is empty
}
// Check if the stack is full
public bool IsFull()
{
return top == items.Length - 1; // Returns true if the stack is full
}
// Push an element onto the stack
public void Push(int item)
{
if (IsFull()) // Check if the stack is full
{
Console.WriteLine("Stack Full!"); // Display a message indicating stack overflow
return;
}
items[++top] = item; // Increment top and add the item to the stack
}
// Pop an element from the stack
public int Pop()
{
if (IsEmpty()) // Check if the stack is empty
{
Console.WriteLine("Stack underflow"); // Display a message indicating stack underflow
return -1;
}
return items[top--]; // Return and decrement top to remove the element from the stack
}
// Peek at the top element of the stack without removing it
public int Peek()
{
if (IsEmpty()) // Check if the stack is empty
{
Console.WriteLine("Stack is empty"); // Display a message indicating the stack is empty
return -1;
}
return items[top]; // Return the top element of the stack
}
// Static method to get the size of a stack
public static int Size(Stack stack)
{
return stack.top + 1; // Return the size of the stack based on the current top index
}
// Method to count all the elements in a stack
public static int Count(Stack stack)
{
int count = 0;
Stack temp = new Stack(Size(stack));
// Move elements from the original stack to a temporary stack and count them
while (!stack.IsEmpty())
{
temp.Push(stack.Pop()); // Move elements from original stack to temporary stack
count++; // Increment count for each element moved
}
// Restore the original stack by moving elements back from the temporary stack
while (!temp.IsEmpty())
{
stack.Push(temp.Pop()); // Move elements back to the original stack
}
return count; // Return the total count of elements in the stack
}
// Check if an element exists in the stack
public bool Contains(int item)
{
for (int i = 0; i <= top; i++)
{
if (items[i] == item)
{
return true; // Return true if the element is found in the stack
}
}
return false; // Return false if the element is not found in the stack
}
// Method to get elements from both stacks without duplicates
public static Stack elements_from_both_stacks_without_duplicates(Stack stack1, Stack stack2)
{
int combinedSize = Size(stack1) + Size(stack2);
Stack result = new Stack(combinedSize);
// Get elements from stack1 without duplicates
for (int i = 0; i <= stack1.top; i++)
{
int item = stack1.items[i];
if (!result.Contains(item))
{
result.Push(item);
}
}
// Get elements from stack2 without duplicates
for (int i = 0; i <= stack2.top; i++)
{
int item = stack2.items[i];
if (!result.Contains(item))
{
result.Push(item);
}
}
return result; // Return the stack containing elements from both stacks without duplicates
}
// Method to display the elements of the stack
public static void Display(Stack stack)
{
if (stack.IsEmpty())
{
Console.WriteLine("Stack is empty");
return;
}
Console.WriteLine("Stack elements:");
for (int i = stack.top; i >= 0; i--)
{
Console.Write(stack.items[i] + " ");
}
}
}
public class Program
{
public static void Main(string[] args)
{
Console.WriteLine("Initialize three stacks:");
// Create and display the first stack
Stack stack1 = new Stack(10);
stack1.Push(1);
stack1.Push(2);
stack1.Push(3);
Console.Write("Stack1: ");
Stack.Display(stack1);
// Create and display the second stack
Stack stack2 = new Stack(10);
stack2.Push(2);
stack2.Push(3);
stack2.Push(4);
stack2.Push(5);
Console.Write("\nStack2: ");
Stack.Display(stack2);
// Create and display the third stack
Stack stack3 = new Stack(10);
stack3.Push(3);
stack3.Push(4);
stack3.Push(5);
Console.Write("\nStack3: ");
Stack.Display(stack3);
// Get elements from stack1 and stack2 without duplicates and display the result
Stack result = Stack.elements_from_both_stacks_without_duplicates(stack1, stack2);
Console.Write("\n\nElements from stack1 and stack2 without duplicates:\n");
Stack.Display(result);
// Get elements from stack2 and stack3 without duplicates and display the result
result = Stack.elements_from_both_stacks_without_duplicates(stack2, stack3);
Console.Write("\n\nElements from stack2 and stack3 without duplicates:\n");
Stack.Display(result);
// Get elements from stack3 and stack1 without duplicates and display the result
result = Stack.elements_from_both_stacks_without_duplicates(stack3, stack1);
Console.Write("\n\nElements from stack3 and stack1 without duplicates:\n");
Stack.Display(result);
}
}
Sample Output:
Initialize three stacks: Stack1: Stack elements: 3 2 1 Stack2: Stack elements: 5 4 3 2 Stack3: Stack elements: 5 4 3 Elements from stack1 and stack2 without duplicates: Stack elements: 5 4 3 2 1 Elements from stack2 and stack3 without duplicates: Stack elements: 5 4 3 2 Elements from stack3 and stack1 without duplicates: Stack elements: 2 1 5 4 3
Flowchart:
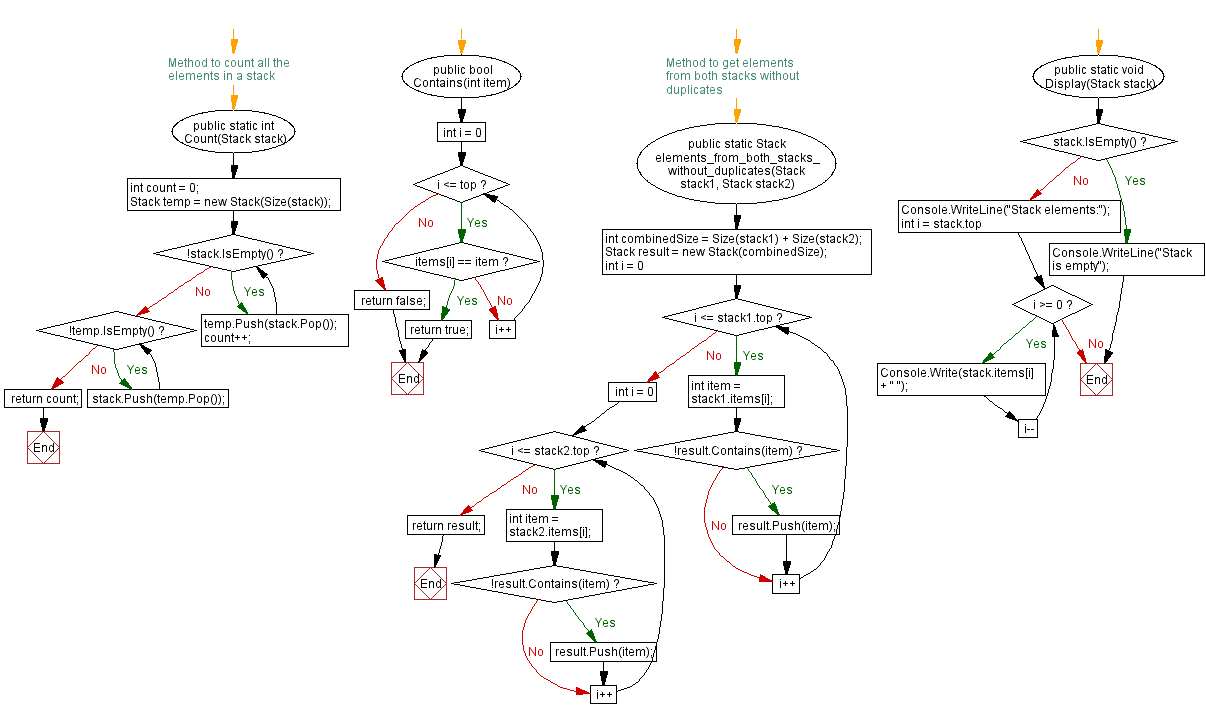
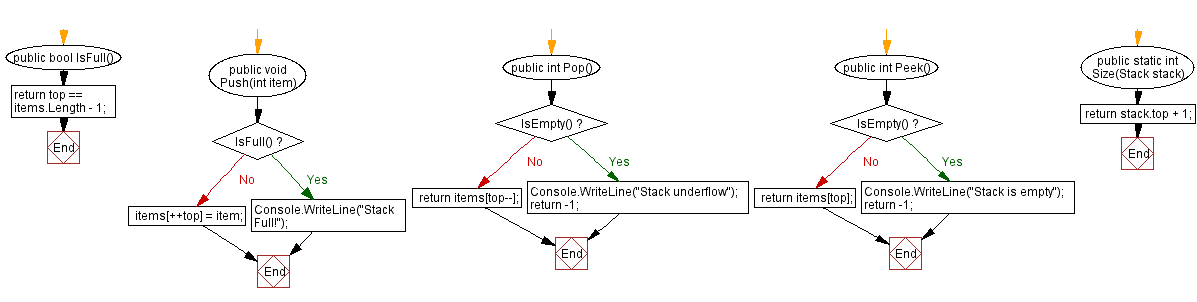
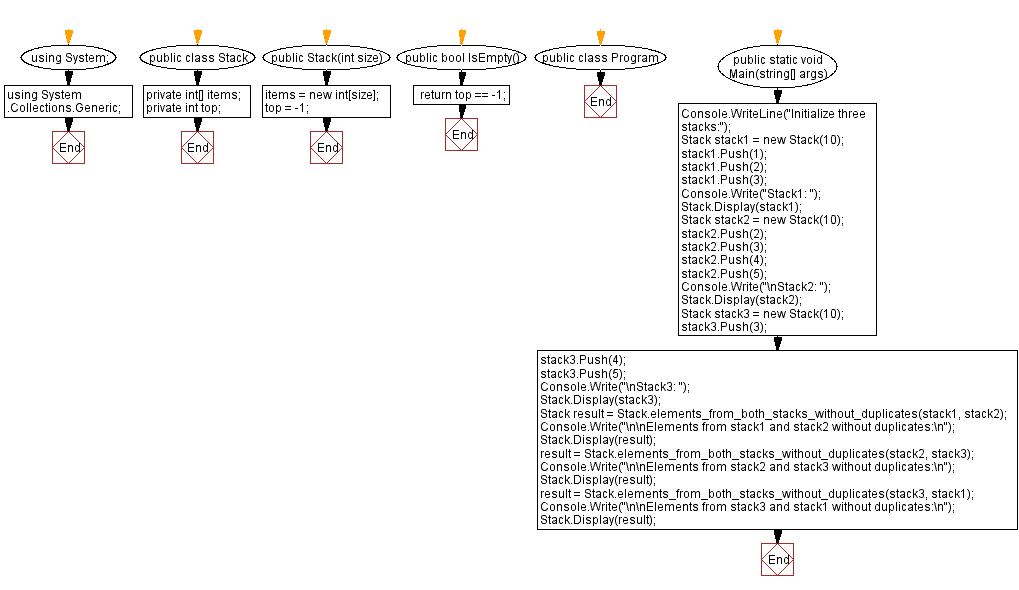
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Stack elements in the first but not in the second.
Next: Symmetric difference of two stacks.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics