C#: Checks if two stacks are equal
Write a C# program that implements a stack and checks if two stacks are equal.
Sample Solution:
C# Code:
using System;
using System.Collections;
using System.Collections.Generic;
public class Stack
{
private int[] items; // Array to hold stack elements
private int top; // Index indicating the top of the stack
// Constructor to initialize the stack with a specified size
public Stack(int size)
{
items = new int[size]; // Creating an array of integers with the given size
top = -1; // Initializing top index to -1 indicating an empty stack
}
// Check if the stack is empty
public bool IsEmpty()
{
return top == -1; // Stack is empty if top is -1
}
// Check if the stack is full
public bool IsFull()
{
return top == items.Length - 1; // Stack is full if top equals the last index of the array
}
// Push an element onto the stack
public void Push(int item)
{
if (IsFull()) // Check if the stack is full
{
Console.WriteLine("Stack Full!"); // Display a message indicating stack overflow
return;
}
items[++top] = item; // Increment top and add the item to the stack
}
// Pop an element from the stack
public int Pop()
{
if (IsEmpty()) // Check if the stack is empty
{
Console.WriteLine("Stack underflow"); // Display a message indicating stack underflow
return -1;
}
return items[top--]; // Return and decrement top to remove the element from the stack
}
// Peek at the top element of the stack without removing it
public int Peek()
{
if (IsEmpty()) // Check if the stack is empty
{
Console.WriteLine("Stack is empty"); // Display a message indicating the stack is empty
return -1;
}
return items[top]; // Return the top element of the stack
}
// Static method to get the size of a stack
public static int Size(Stack stack)
{
return stack.top + 1; // Return the size of the stack based on the current top index
}
// Method to count all the elements in a stack
public static int Count(Stack stack)
{
int count = 0; // Initialize count variable to track the number of elements
Stack temp = new Stack(Size(stack)); // Create a temporary stack to preserve the original stack order
// Move elements from the original stack to the temporary stack and count them
while (!stack.IsEmpty())
{
temp.Push(stack.Pop()); // Move elements from original stack to temporary stack
count++; // Increment count for each element moved
}
// Restore the original stack by moving elements back from the temporary stack
while (!temp.IsEmpty())
{
stack.Push(temp.Pop()); // Move elements back to the original stack
}
return count; // Return the total count of elements in the stack
}
// Static method to clear the stack by popping all elements
public static void Clear(Stack stack)
{
while (!stack.IsEmpty())
{
stack.Pop(); // Remove elements from the stack until it's empty
}
}
// Method to check if an element is present or not in a stack
public static bool Contains(Stack stack, int item)
{
if (stack == null || stack.IsEmpty())
return false;
bool found = false;
Stack tempStack = new Stack(Size(stack));
while (!stack.IsEmpty())
{
int current = stack.Pop();
if (current == item)
{
found = true;
break;
}
tempStack.Push(current);
}
while (!tempStack.IsEmpty())
{
stack.Push(tempStack.Pop());
}
return found;
}
// Check if two stacks are equal or not
public static bool AreStacksEqual(Stack stack1, Stack stack2)
{
if (stack1 == null || stack2 == null)
{
return false;
}
if (Count(stack1) != Count(stack2))
{
return false;
}
Stack temp1 = new Stack(Count(stack1));
Stack temp2 = new Stack(Count(stack2));
while (Count(temp1) > 0 && Count(temp2) > 0)
{
if (!temp1.Pop().Equals(temp2.Pop()))
{
return false;
}
}
return true;
}
// Method to display the elements of the stack
public static void Display(Stack stack)
{
if (stack.IsEmpty())
{
Console.WriteLine("Stack is empty");
return;
}
Console.WriteLine("Stack elements:");
for (int i = stack.top; i >= 0; i--)
{
Console.Write(stack.items[i] + " ");
}
}
}
public class Program
{
public static void Main(string[] args)
{
Console.WriteLine("Initialize a stack:");
Stack stack1 = new Stack(6);
stack1.Push(1);
stack1.Push(2);
stack1.Push(3);
stack1.Push(4);
stack1.Push(5);
stack1.Push(6);
Console.WriteLine("\nStack-1");
Stack.Display(stack1);
Stack stack2 = new Stack(3);
stack2.Push(1);
stack2.Push(2);
stack2.Push(3);
Console.WriteLine("\nStack-2");
Stack.Display(stack2);
Stack stack3 = new Stack(3);
stack3.Push(1);
stack3.Push(2);
stack3.Push(3);
Console.WriteLine("\nStack-3");
Stack.Display(stack3);
Console.WriteLine("\nIf stack2 is equal of stack3!");
bool result = Stack.AreStacksEqual(stack2, stack3);
Console.WriteLine("{0}", result);
Console.WriteLine("\nIf stack1 is equal of stack3!");
result = Stack.AreStacksEqual(stack1, stack3);
Console.WriteLine("{0}", result);
Console.WriteLine("\nIf stack1 is equal of stack2!");
result = Stack.AreStacksEqual(stack1, stack2);
Console.WriteLine("{0}", result);
}
}
Sample Output:
Initialize a stack: Stack-1 Stack elements: 6 5 4 3 2 1 Stack-2 Stack elements: 3 2 1 Stack-3 Stack elements: 3 2 1 If stack2 is equal of stack3! True If stack1 is equal of stack3! False If stack1 is equal of stack2! False
Flowchart:
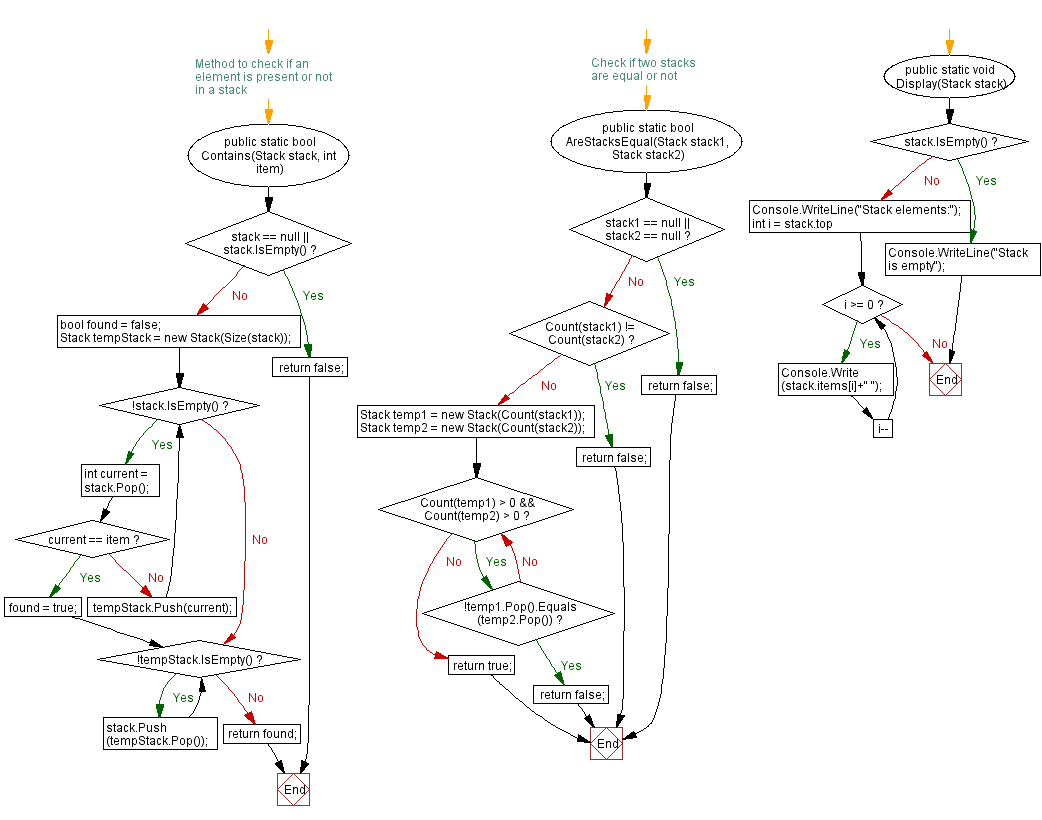
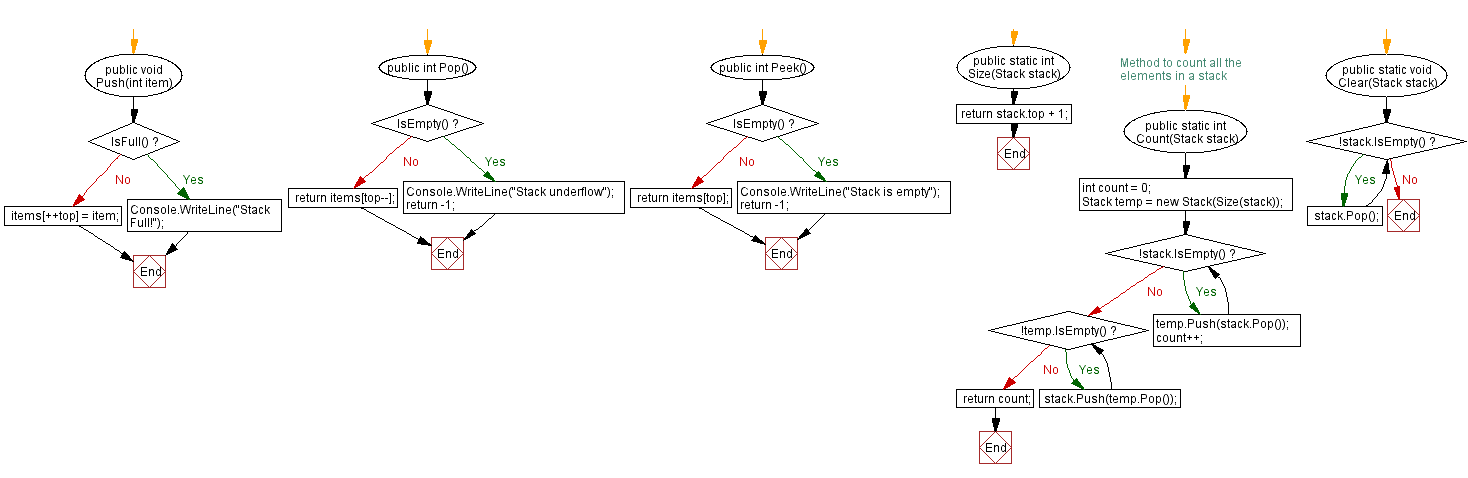
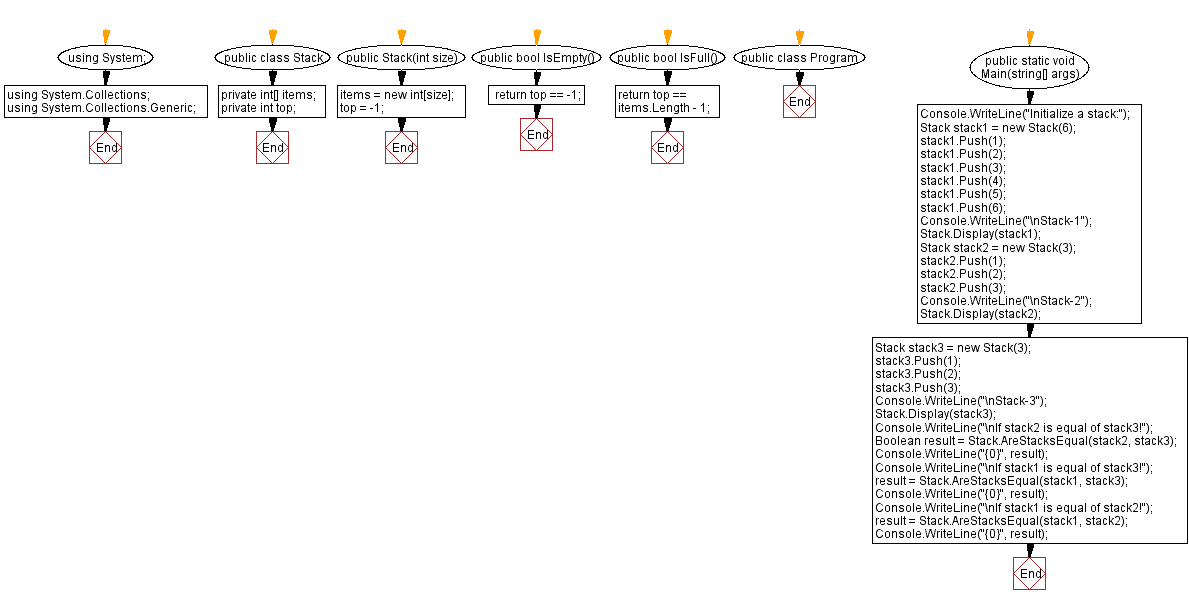
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Check if a stack is a subset of another stack.
Next: Find common elements between two stacks.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics