C#: Merge two stacks into one
Write a C# program to merge two stacks into one.
Sample Solution:
C# Code:
using System;
using System.Collections;
using System.Collections.Generic;
public class Stack
{
private int[] items; // Array to hold stack elements
private int top; // Index indicating the top of the stack
// Constructor to initialize the stack with a specified size
public Stack(int size)
{
items = new int[size]; // Creating an array of integers with the given size
top = -1; // Initializing top index to -1 indicating an empty stack
}
// Check if the stack is empty
public bool IsEmpty()
{
return top == -1; // Stack is empty if top is -1
}
// Check if the stack is full
public bool IsFull()
{
return top == items.Length - 1; // Stack is full if top equals the last index of the array
}
// Push an element onto the stack
public void Push(int item)
{
if (IsFull()) // Check if the stack is full
{
Console.WriteLine("Stack Full!"); // Display a message indicating stack overflow
return;
}
items[++top] = item; // Increment top and add the item to the stack
}
// Pop an element from the stack
public int Pop()
{
if (IsEmpty()) // Check if the stack is empty
{
Console.WriteLine("Stack underflow"); // Display a message indicating stack underflow
return -1;
}
return items[top--]; // Return and decrement top to remove the element from the stack
}
// Peek at the top element of the stack without removing it
public int Peek()
{
if (IsEmpty()) // Check if the stack is empty
{
Console.WriteLine("Stack is empty"); // Display a message indicating the stack is empty
return -1;
}
return items[top]; // Return the top element of the stack
}
// Static method to get the size of a stack
public static int Size(Stack stack)
{
return stack.top + 1; // Return the size of the stack based on the current top index
}
// Static method to count all the elements in a stack
public static int Count(Stack stack)
{
int count = 0; // Initialize count variable to track the number of elements
Stack temp = new Stack(Size(stack)); // Create a temporary stack to preserve the original stack order
// Move elements from the original stack to the temporary stack and count them
while (!stack.IsEmpty())
{
temp.Push(stack.Pop()); // Move elements from original stack to temporary stack
count++; // Increment count for each element moved
}
// Restore the original stack by moving elements back from the temporary stack
while (!temp.IsEmpty())
{
stack.Push(temp.Pop()); // Move elements back to the original stack
}
return count; // Return the total count of elements in the stack
}
// Static method to clear the stack by popping all elements
public static void Clear(Stack stack)
{
while (!stack.IsEmpty())
{
stack.Pop(); // Remove elements from the stack until it's empty
}
}
// Method to merge two stacks
public static Stack MergeStacks(Stack stack1, Stack stack2)
{
Stack result = new Stack(Size(stack1) + Size(stack2)); // Create a new stack to hold merged elements
// Push elements from stack1 to the result stack
while (!stack1.IsEmpty())
{
result.Push(stack1.Pop());
}
// Push elements from stack2 to the result stack
while (!stack2.IsEmpty())
{
result.Push(stack2.Pop());
}
return result; // Return the merged stack
}
// Method to display the elements of the stack
public static void Display(Stack stack)
{
if (stack.IsEmpty()) // Check if the stack is empty
{
Console.WriteLine("Stack is empty"); // Display a message indicating the stack is empty
return;
}
Console.WriteLine("\nStack elements:"); // Display a message indicating stack elements will be shown
for (int i = stack.top; i >= 0; i--) // Loop through the stack elements
{
Console.Write(stack.items[i] + " "); // Print each element of the stack
}
}
}
public class Program
{
public static void Main(string[] args)
{
Console.WriteLine("Initialize a stack:");
Stack stack = new Stack(10); // Create a stack with a capacity of 10 elements
Console.WriteLine("Input some elements onto the stack:");
Stack stack1 = new Stack(3); // Create stack1 with a capacity of 3 elements
stack1.Push(1); // Add elements to stack1
stack1.Push(2);
stack1.Push(3);
Stack.Display(stack1); // Display elements of stack1
Stack stack2 = new Stack(3); // Create stack2 with a capacity of 3 elements
stack2.Push(4); // Add elements to stack2
stack2.Push(5);
stack2.Push(6);
Stack.Display(stack2); // Display elements of stack2
Console.WriteLine("\n\nMerged stacks:");
Stack mergedStack = Stack.MergeStacks(stack1, stack2); // Merge stack1 and stack2 into mergedStack
Stack.Display(mergedStack); // Display elements of mergedStack
}
}
Sample Output:
Initialize a stack: Input some elements onto the stack: Stack elements: 3 2 1 Stack elements: 6 5 4 Merged stacks: Stack elements: 4 5 6 1 2 3
Flowchart:
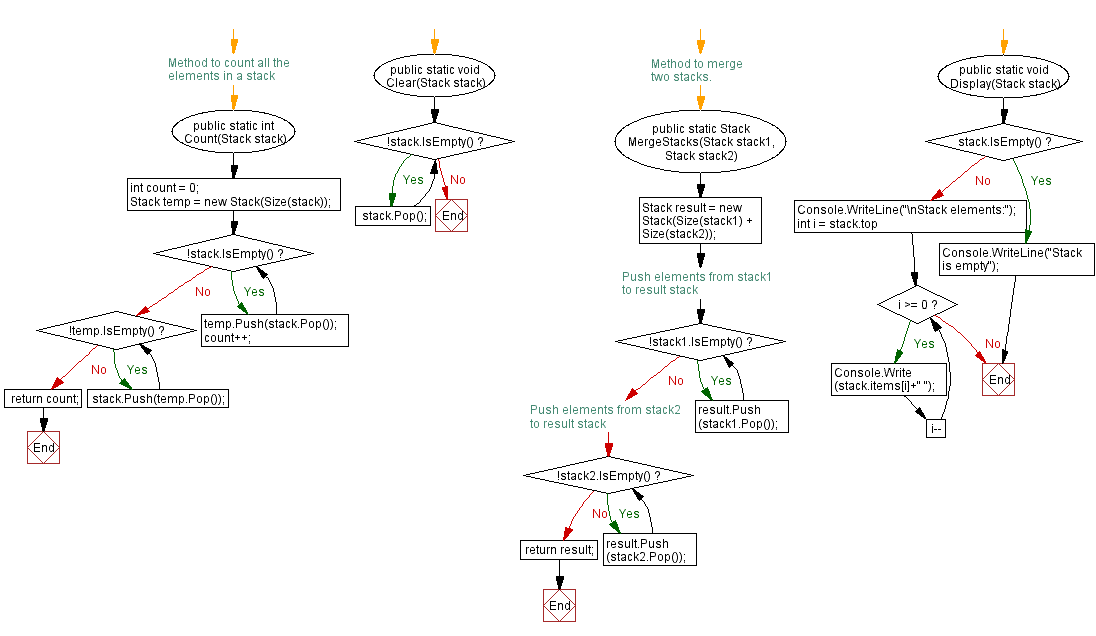
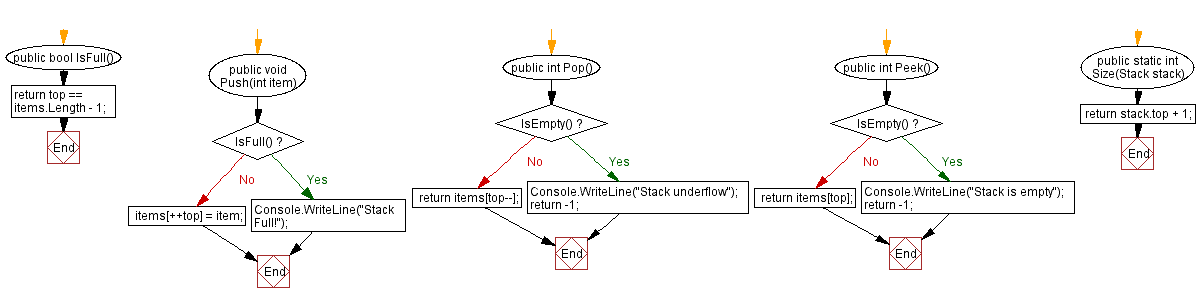
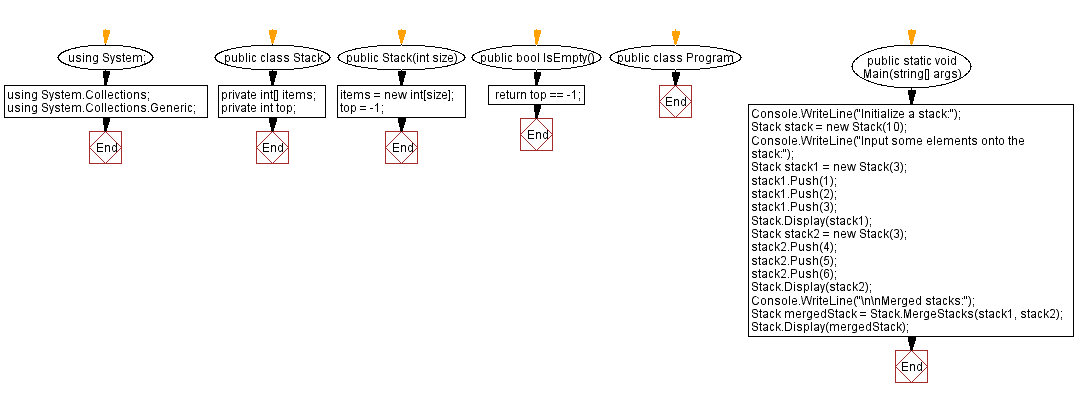
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Nth element from the top of the stack.
Next: Check if a stack is a subset of another stack.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics