C#: Nth element from the top of the stack
C# Sharp Stack: Exercise-16 with Solution
Write a C# program to get the nth element from the top of the stack.
Sample Solution:
C# Code:
// Using directives for necessary namespaces
using System;
using System.Collections;
using System.Collections.Generic;
// Definition of the Stack class
public class Stack
{
// Private fields for stack implementation
private int[] items;
private int top;
// Constructor to initialize the stack with a specified size
public Stack(int size)
{
items = new int[size];
top = -1;
}
// Method to check if the stack is empty
public bool IsEmpty()
{
return top == -1;
}
// Method to check if the stack is full
public bool IsFull()
{
return top == items.Length - 1;
}
// Method to push an item onto the stack
public void Push(int item)
{
// Check if the stack is full before pushing
if (IsFull())
{
Console.WriteLine("Stack Full!");
return;
}
// Increment the top index and push the item
items[++top] = item;
}
// Method to pop an item from the stack
public int Pop()
{
// Check if the stack is empty before popping
if (IsEmpty())
{
Console.WriteLine("Stack underflow");
return -1;
}
// Return the popped item and decrement the top index
return items[top--];
}
// Method to peek at the top item without removing it
public int Peek()
{
if (IsEmpty())
{
Console.WriteLine("Stack is empty");
return -1;
}
return items[top];
}
// Static method to get the size of a stack
public static int Size(Stack stack)
{
return stack.top + 1;
}
// Static method to count all the elements in a stack
public static int Count(Stack stack)
{
int count = 0;
// Create a temporary stack to preserve original stack elements
Stack temp = new Stack(Size(stack));
// Transfer elements to the temporary stack while counting
while (!stack.IsEmpty())
{
temp.Push(stack.Pop());
count++;
}
// Restore the original stack
while (!temp.IsEmpty())
{
stack.Push(temp.Pop());
}
return count;
}
// Static method to clear all elements from a stack
public static void Clear(Stack stack)
{
while (!stack.IsEmpty())
{
stack.Pop();
}
}
// Static method to get the Nth element from the top of the stack
public static int GetNthFromTop(Stack stack, int n)
{
// Check if there are enough elements in the stack
if (Count(stack) < n)
{
throw new ArgumentException("Not enough elements!");
}
// Create a temporary stack to store popped elements
Stack<int> tempStack = new Stack<int>();
// Pop the first N elements from the original stack
for (int i = 0; i < n; i++)
{
tempStack.Push(stack.Pop());
}
// Peek at the Nth element
int result = tempStack.Peek();
// Restore the popped elements to the original stack
while (tempStack.Count > 0)
{
stack.Push(tempStack.Pop());
}
return result;
}
// Static method to display the elements of a stack
public static void Display(Stack stack)
{
if (stack.IsEmpty())
{
Console.WriteLine("Stack is empty");
return;
}
Console.WriteLine("Stack elements:");
// Display elements in reverse order (from top to bottom)
for (int i = stack.top; i >= 0; i--)
{
Console.Write(stack.items[i] + " ");
}
}
}
// Main program class
public class Program
{
public static void Main(string[] args)
{
// Initialize a stack with a specified size
Console.WriteLine("Initialize a stack:");
Stack stack = new Stack(10);
// Push some elements onto the stack
Console.WriteLine("Input some elements onto the stack:");
stack.Push(1);
stack.Push(2);
stack.Push(3);
stack.Push(4);
stack.Push(5);
stack.Push(6);
// Display the current state of the stack
Stack.Display(stack);
// Get and display the Nth element from the top of the stack
int n = 2;
int el = Stack.GetNthFromTop(stack, n);
Console.WriteLine("\n\nNth element from the top of the stack where n = {0} is {1}", n, el);
// Further examples of getting and displaying Nth elements
n = 3;
el = Stack.GetNthFromTop(stack, n);
Stack.Display(stack);
Console.WriteLine("\nNth element from the top of the stack where n = {0} is {1}", n, el);
n = 4;
el = Stack.GetNthFromTop(stack, n);
Stack.Display(stack);
Console.WriteLine("\nNth element from the top of the stack where n = {0} is {1}", n, el);
n = 6;
el = Stack.GetNthFromTop(stack, n);
Stack.Display(stack);
Console.WriteLine("\nNth element from the top of the stack where n = {0} is {1}", n, el);
}
}
Sample Output:
Initialize a stack: Input some elements onto the stack: Stack elements: 6 5 4 3 2 1 Nth element from the top of the stack where n = 2 is 5 Stack elements: 6 5 4 3 2 1 Nth element from the top of the stack where n = 3 is 4 Stack elements: 6 5 4 3 2 1 Nth element from the top of the stack where n = 4 is 3 Stack elements: 6 5 4 3 2 1 Nth element from the top of the stack where n = 6 is 1
Flowchart:
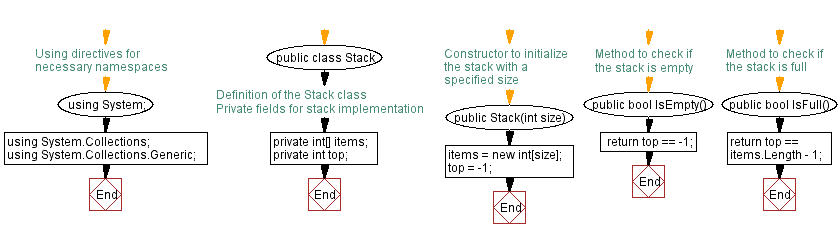
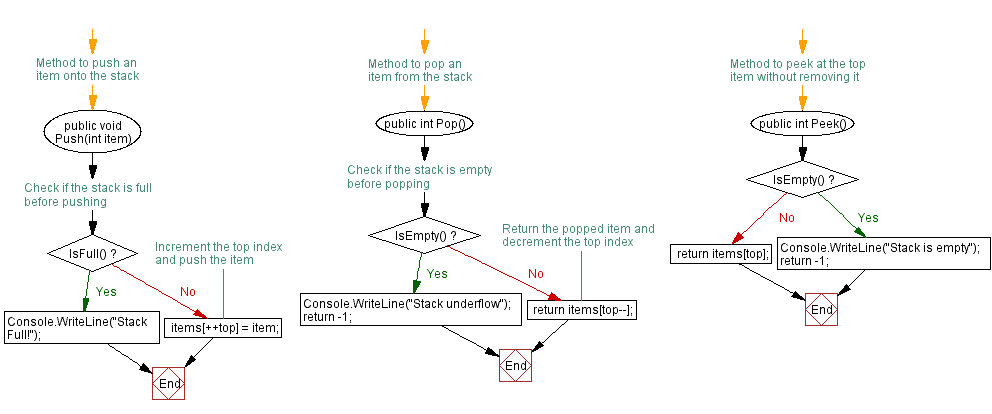
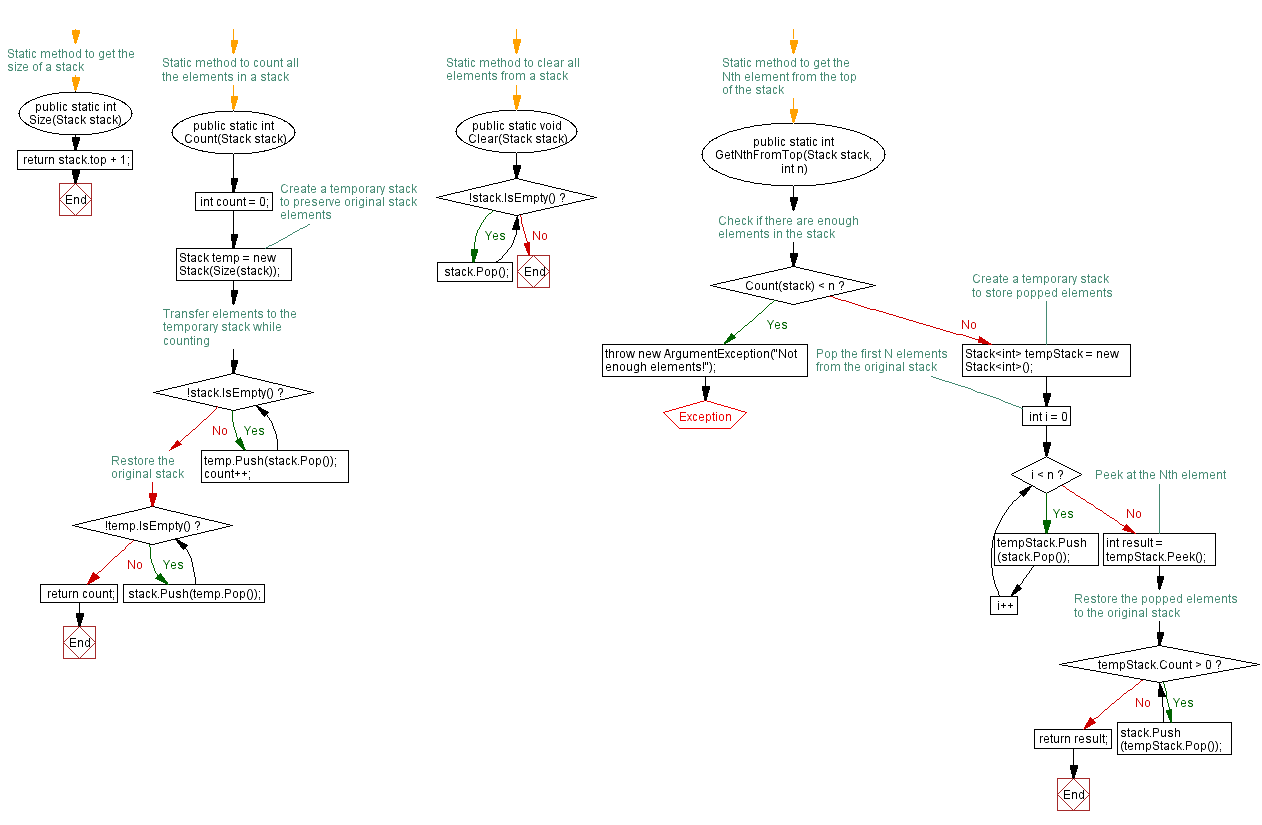
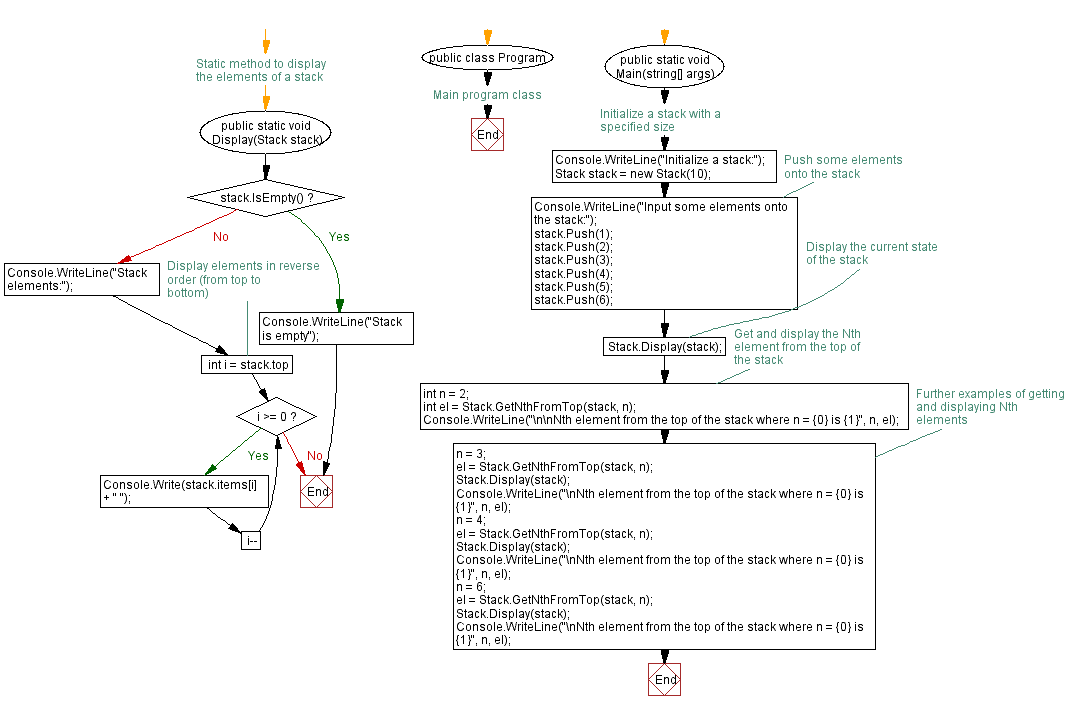
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Swap the top two elements of a stack.
Next: Merge two stacks into one.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/csharp-exercises/stack/csharp-stack-exercise-16.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics