C#: Find the factorial of a given number
Write a program in C# Sharp to find the factorial of a given number using recursion.
Visual Presentation:
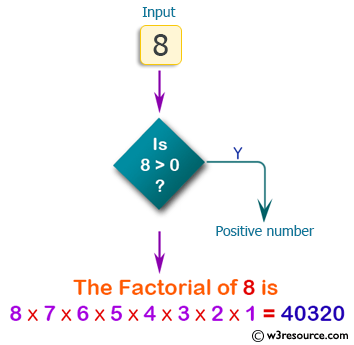
Sample Solution:
C# Sharp Code:
using System;
class RecExercise9
{
// Main method: entry point of the program
static void Main(string[] args)
{
Console.WriteLine("\n\n Recursion : Find the factorial of a given number :");
Console.WriteLine("-------------------------------------------------------");
// Accept user input for the number
Console.Write(" Input any positive number : ");
int n1 = Convert.ToInt32(Console.ReadLine());
// Call the FactorialCalcu method and store the result in 'fact'
long fact = FactorialCalcu(n1);
// Display the factorial of the input number
Console.WriteLine(" The factorial of {0} is : {1} ", n1, fact);
Console.ReadKey(); // Wait for a key press to exit
}
// Recursive method to calculate factorial of a number
private static long FactorialCalcu(int n1)
{
// Base case: if n1 is 0, return 1 (base factorial condition)
if (n1 == 0)
{
return 1;
}
// Recursive call: Multiply n1 with factorial of n1-1 until reaching the base case
return n1 * FactorialCalcu(n1 - 1);
}
}
Sample Output:
Recursion : Find the factorial of a given number : ------------------------------------------------------- Input any positive number : 8 The factorial of 8 is : 40320
Flowchart :
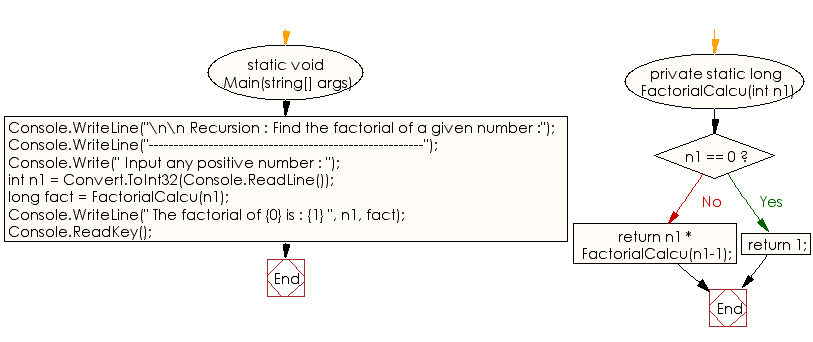
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a program in C# Sharp to Check whether a given String is Palindrome or not using recursion.
Next: Write a program in C# Sharp to find the Fibonacci numbers for a n numbers of series using recursion.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics