C#: Check whether a string is Palindrome or not
Write a program in C# Sharp to check whether a given string is a palindrome or not using recursion.
Visual Presentation:
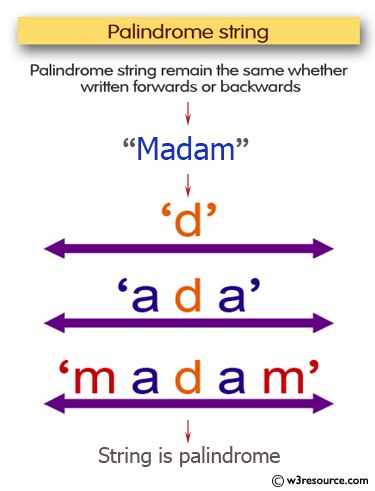
Sample Solution:
C# Sharp Code:
using System;
public class RecExercise8
{
// Method to check if a string is a palindrome using recursion
public static bool IsPalindrome(string text)
{
// Base case: If the length of the text is 0 or 1, it's a palindrome
if (text.Length <= 1)
return true;
else
{
// Check if the first and last characters are equal
if (text[0] != text[text.Length - 1])
return false; // If not equal, it's not a palindrome
else
// Recursive call: Check the substring without the first and last characters
return IsPalindrome(text.Substring(1, text.Length - 2));
}
}
public static void Main()
{
Console.Write("\n\n Recursion : Check whether a string is a Palindrome or not :\n");
Console.Write("---------------------------------------------------------------\n");
string str1;
bool tf;
Console.Write(" Input a string : ");
str1 = Console.ReadLine();
// Call IsPalindrome function and get the result
tf = IsPalindrome(str1);
// Output whether the input string is a palindrome or not
if (tf == true)
{
Console.WriteLine(" The string is a Palindrome.\n");
}
else
{
Console.WriteLine(" The string is not a Palindrome.\n");
}
}
}
Sample Output:
Recursion : Check whether a string ia Palindrome or not : --------------------------------------------------------------- Input a string : eye The string is Palindrome.
Flowchart :
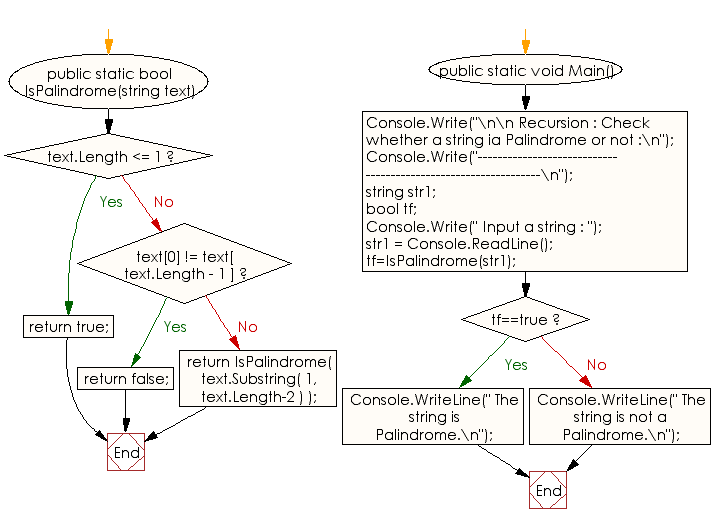
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a program in C# Sharp to check whether a number is prime or not using recursion.
Next: Write a program in C# Sharp to find the factorial of a given number using recursion.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.