C#: Count the number of digits in a number
Write a program in C# Sharp to count the number of digits in a number using recursion.
Visual Presentation:
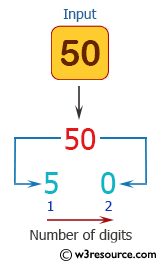
Sample Solution:
C# Sharp Code:
using System;
// Class definition named 'RecExercise5'
class RecExercise5
{
// Main method, the entry point of the program
static void Main(string[] args)
{
// Display a description of the program
Console.Write("\n\n Recursion : Count the number of digits in a number :\n");
Console.Write("---------------------------------------------------------\n");
Console.Write(" Input any number : ");
int num = Convert.ToInt32(Console.ReadLine()); // Read user input for the number
// Display the number of digits in the entered number
Console.Write("\n The number {0} contains number of digits : {1} ", num, getDigits(num, 0));
Console.ReadLine();
}
// Recursive function to count the number of digits in a number
public static int getDigits(int n1, int nodigits)
{
// Base case: if the number becomes zero, return the count of digits
if (n1 == 0)
return nodigits;
// Recursive call: getDigits method is called with the quotient of the number divided by 10 and the incremented count of digits
return getDigits(n1 / 10, ++nodigits);
}
}
Sample Output:
Recursion : Count the number of digits in a number : --------------------------------------------------------- Input any number : 1000 The number 1000 contains number of digits : 4
Flowchart :
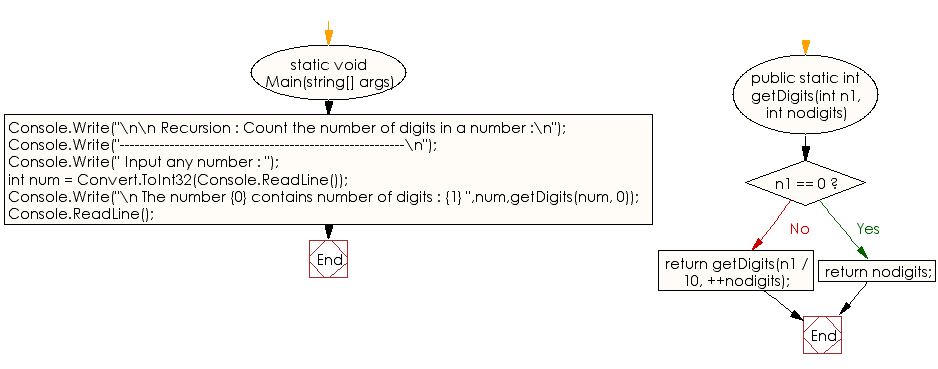
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a program in C# Sharp to display the individual digits of a given number using recursion.
Next: Write a program in C# Sharp to print even or odd numbers in a given range using recursion.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.