C#: Display the individual digits of a given number
C# Sharp Recursion: Exercise-4 with Solution
Write a program in C# Sharp to display the individual digits of a given number using recursion.
Visual Presentation:
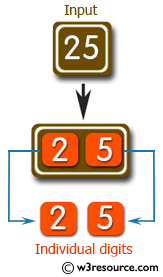
Sample Solution:
C# Sharp Code:
using System;
// Class definition named 'RecExercise4'
public class RecExercise4
{
// Main method, the entry point of the program
static void Main()
{
// Display a description of the program
Console.Write("\n\n Recursion : Display the individual digits of a given number :\n");
Console.Write("------------------------------------------------------------------\n");
Console.Write(" Input any number : ");
int num = Convert.ToInt32(Console.ReadLine()); // Read user input for the number
// Display the message indicating the digits in the entered number
Console.Write(" The digits in the number {0} are : ", num);
// Call a recursive function to separate and display the individual digits of the entered number
separateDigits(num);
Console.Write("\n\n");
}
// Recursive function to separate and display individual digits of a number
static void separateDigits(int n)
{
// Base case: if the number has only one digit
if (n < 10)
{
Console.Write("{0} ", n); // Display the single digit
return;
}
// Recursive call: separateDigits method is called for the quotient of the number divided by 10
separateDigits(n / 10);
Console.Write(" {0} ", n % 10); // Display the remainder (last digit)
}
}
Sample Output:
Recursion : Display the individual digits of a given number : ------------------------------------------------------------------ Input any number : 25 The digits in the number 25 are : 2 5
Flowchart:
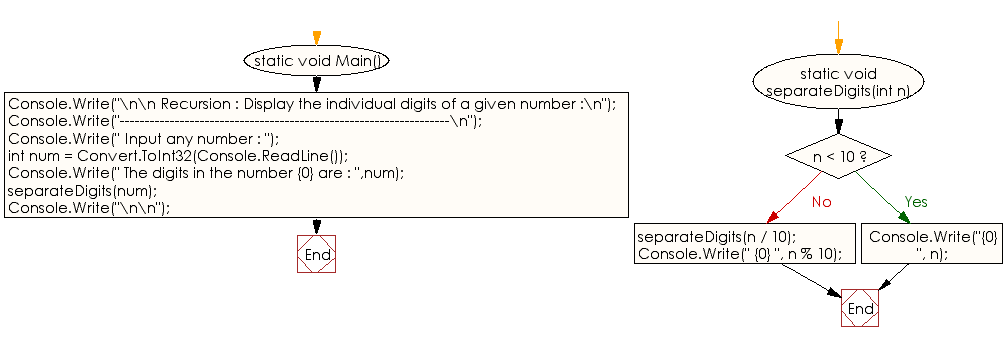
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a program in C# Sharp to find the sum of first n natural numbers using recursion.
Next: Write a program in C# Sharp to count the number of digits in a number using recursion.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/csharp-exercises/recursion/csharp-recursion-exercise-4.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics