C#: Sum of first n natural numbers
C# Sharp Recursion : Exercise-3 with Solution
Write a program in C# Sharp to find the sum of the first n natural numbers using recursion.
Visual Presentation:
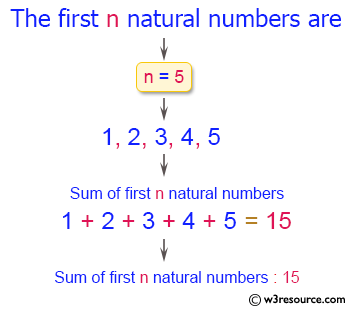
Sample Solution:
C# Sharp Code:
using System;
// Class definition named 'RecExercise3'
class RecExercise3
{
// Main method, the entry point of the program
static void Main(string[] args)
{
// Display a description of the program
Console.Write("\n\n Recursion : Sum of first n natural numbers :\n");
Console.Write("--------------------------------------------------\n");
Console.Write(" How many numbers to sum : ");
int n = Convert.ToInt32(Console.ReadLine()); // Read the user input for the count of numbers to sum
// Calculate and display the sum of the first 'n' natural numbers
Console.Write(" The sum of first {0} natural numbers is : {1}\n\n", n, SumOfTen(1, n));
}
// Function to initiate the calculation of the sum
static int SumOfTen(int min, int max)
{
return CalcuSum(min, max);
}
// Recursive function to calculate the sum of natural numbers from 'min' to 'val'
static int CalcuSum(int min, int val)
{
// Base case: if the current value is equal to the minimum value, return the value itself
if (val == min)
return val;
// Recursive call: Add the current value with the sum of previous values from 'min' to 'val-1'
return val + CalcuSum(min, val - 1);
}
}
Sample Output:
Recursion : Sum of first n natural numbers : -------------------------------------------------- How many numbers to sum : 5 The sum of first 5 natural numbers is : 15
Flowchart :
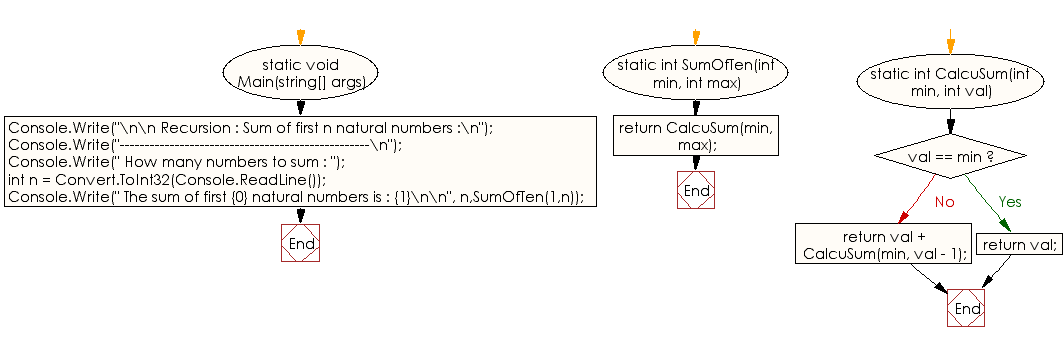
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a program in C# Sharp to print numbers from n to 1 using recursion.
Next: Write a program in C# Sharp to display the individual digits of a given number using recursion.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/csharp-exercises/recursion/csharp-recursion-exercise-3.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics