C#: Print the natural numbers from n to 1
Write a program in C# Sharp to print numbers from n to 1 using recursion.
Visual Presentation:
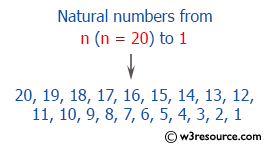
Sample Solution:
C# Sharp Code:
using System;
// Class definition named 'RecExercise1'
class RecExercise1
{
// Recursive method to print natural numbers from 'ctr' to 1
static int printNatural(int ctr, int stval)
{
// Base case: If the counter becomes less than 1, return the starting value
if (ctr < 1)
{
return stval;
}
Console.Write(" {0} ", ctr); // Print the current number
ctr--; // Decrement the counter
// Recursive call: Decrement 'ctr' and continue printing natural numbers
return printNatural(ctr, stval);
}
// Main method, the entry point of the program
static void Main()
{
// Display a description of the program
Console.Write("\n\n Recursion : Print the natural numbers from n to 1 :\n");
Console.Write("--------------------------------------------------------\n");
Console.Write(" How many numbers to print : ");
int ctr = Convert.ToInt32(Console.ReadLine()); // Read the user input for the count of numbers
// Call the recursive method to print natural numbers from 'ctr' to 1
printNatural(ctr, 1);
Console.Write("\n\n");
}
}
Sample Output:
Recursion : Print the natural numbers from n to 1 : -------------------------------------------------------- How many numbers to print : 20 20 19 18 17 16 15 14 13 12 11 10 9 8 7 6 5 4 3 2 1
Flowchart :
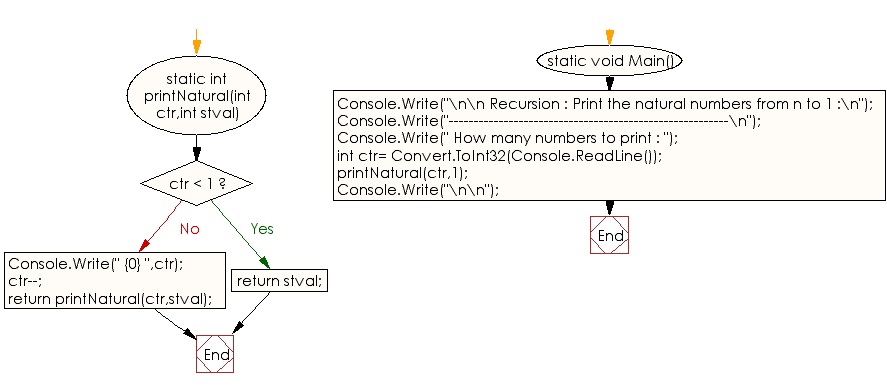
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a program in C# Sharp to print the first n natural number using recursion.
Next: Write a program in C# Sharp to find the sum of first n natural numbers using recursion.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.