C#: Calculate power of any number
Write a program in C# Sharp to calculate the power of any number using recursion.
Visual Presentation:
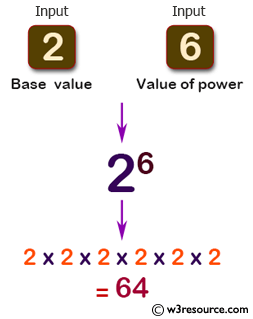
Sample Solution:
C# Sharp Code:
using System;
public class RecExercise15
{
public static void Main()
{
// Declaring variables for base number, exponent, and result
int bNum, pwr, result;
// Prompting the user for the base value
Console.Write("\n\n Recursion : Calculate power of any number :\n");
Console.Write("------------------------------------------------\n");
Console.Write(" Input the base value : ");
bNum = Convert.ToInt32(Console.ReadLine());
// Prompting the user for the exponent
Console.Write(" Input the exponent : ");
pwr = Convert.ToInt32(Console.ReadLine());
// Calling the function to calculate the power
result = CalcuOfPower(bNum, pwr);
// Displaying the result
Console.Write(" The value of {0} to the power of {1} is : {2} \n\n", bNum, pwr, result);
}
// Recursive function to calculate power
public static int CalcuOfPower(int x, int y)
{
// Base case: when the exponent is 0, return 1
if (y == 0)
return 1;
else
// Recursive case: multiply base number x by itself (y - 1) times
return x * CalcuOfPower(x, y - 1);
}
}
Sample Output:
Recursion : Calculate power of any number : ------------------------------------------------ Input the base value : 2 Input the exponent : 6 The value of 2 to the power of 6 is : 64
Flowchart :
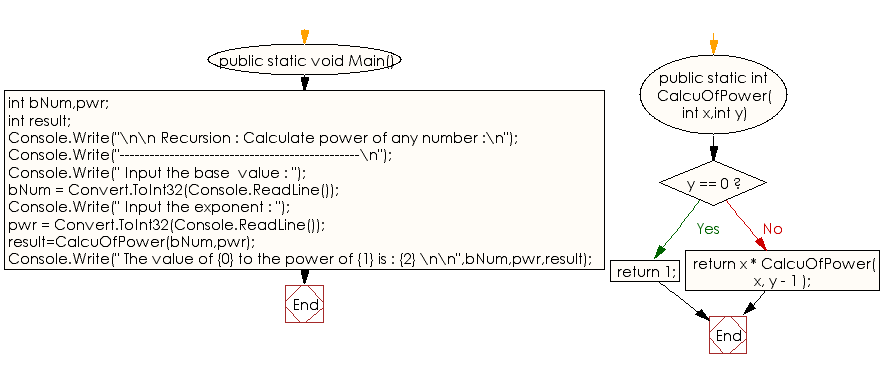
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a program in C# Sharp to get the reverse of a string using recursion.
Next: C# Sharp LINQ Exercises.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.