C#: Find the LCM and GCD of two numbers
Write a program in C# Sharp to find the LCM and GCD of two numbers using recursion.
Visual Presentation:
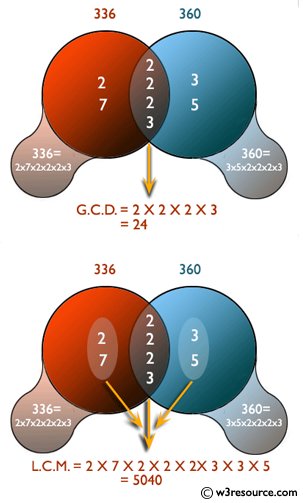
Sample Solution:
C# Sharp Code:
using System;
using System.Text;
// Class RecExercise12 for finding GCD and LCM of two numbers
class RecExercise12
{
// Main method to execute the program
public static void Main()
{
// Variables to store input numbers, GCD, and LCM
long num1, num2, hcf, lcm;
// Prompt for and accept user input of two numbers
Console.WriteLine("\n\n Recursion : Find the LCM and GCD of two numbers :");
Console.WriteLine("------------------------------------------------------");
Console.Write(" Input the first number : ");
num1 = Convert.ToInt64(Console.ReadLine());
Console.Write(" Input the second number : ");
num2 = Convert.ToInt64(Console.ReadLine());
// Calculate GCD and LCM using respective methods
hcf = gcd(num1, num2);
lcm = (num1 * num2) / hcf;
// Display the calculated GCD and LCM
Console.WriteLine("\n The GCD of {0} and {1} = {2} ", num1, num2, hcf);
Console.WriteLine(" The LCM of {0} and {1} = {2}\n", num1, num2, lcm);
}
// Method to calculate the Greatest Common Divisor (GCD) using recursion
static long gcd(long n1, long n2)
{
if (n2 == 0)
{
return n1;
}
else
{
return gcd(n2, n1 % n2); // Recursive call to find GCD
}
}
}
Sample Output:
Recursion : Find the LCM and GCD of two numbers : ------------------------------------------------------ Input the first number : 2 Input the second number : 5 The GCD of 2 and 5 = 1 The LCM of 2 and 5 = 10
Flowchart :
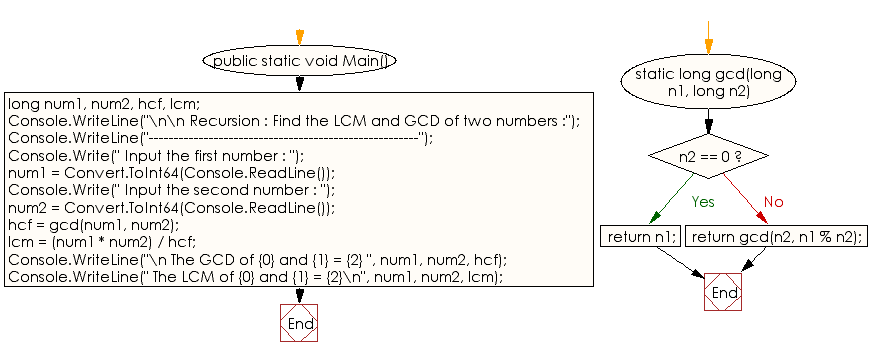
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a program in C# Sharp to generate all possible permutations of an array using recursion.
Next: Write a program in C# Sharp to convert a decimal number to binary using recursion.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.