C#: Find the Fibonacci numbers for a n numbers of series
C# Sharp Recursion: Exercise-10 with Solution
Write a program in C# Sharp to find the Fibonacci numbers for a series of n numbers using recursion.
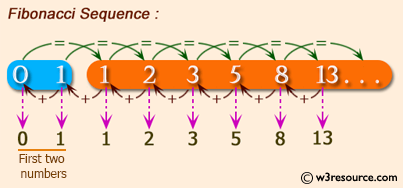
Sample Solution:-
C# Sharp Code:
using System;
class RecExercise10
{
// Method to find Fibonacci number at a specific position 'n'
public static int FindFibonacci(int n)
{
// Initializing Fibonacci sequence variables
int p = 0; // Initializing first number of the series
int q = 1; // Initializing second number of the series
// Loop to calculate Fibonacci series up to the 'n-th' term
for (int i = 0; i < n; i++)
{
int temp = p; // Storing the value of the first number in temporary variable
p = q; // Assigning the value of the second number to the first
q = temp + q; // Calculating the next number in the series
}
return p; // Returning the 'n-th' Fibonacci number
}
// Main method: Entry point of the program
static void Main()
{
Console.WriteLine("\n\n Recursion : Find the Fibonacci numbers for a n numbers of series :");
Console.WriteLine("-----------------------------------------------------------------------");
// Accept user input for the number of terms in the Fibonacci series
Console.Write(" Input number of terms for the Fibonacci series : ");
int n1 = Convert.ToInt32(Console.ReadLine());
Console.Write("\n The Fibonacci series of {0} terms is : ", n1);
// Loop to print the Fibonacci series up to 'n1' terms
for (int i = 0; i < n1; i++)
{
Console.Write("{0} ", FindFibonacci(i)); // Calling FindFibonacci method to find each number
}
Console.ReadKey(); // Wait for a key press to exit
}
}
Sample Output:
Recursion : Find the Fibonacci numbers for a n numbers of series : ----------------------------------------------------------------------- Input number of terms for the Fibonacci series : 10 The Fibonacci series of 10 terms is : 0 1 1 2 3 5 8 13 21 34
Flowchart :
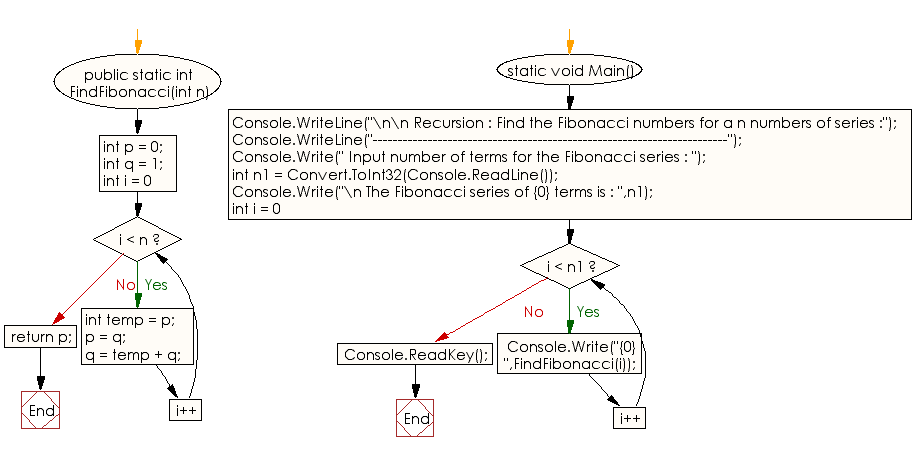
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a program in C# Sharp to find the factorial of a given number using recursion.
Next: Write a program in C# Sharp to generate all possible permutations of an array using recursion.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/csharp-exercises/recursion/csharp-recursion-exercise-10.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics