C# Sharp Exercises: Find a specific word in a string
C# Sharp Regular Expression: Exercise-9 with Solution
Write a C# Sharp program to locate the word “C#” in a given string. If the word "C#" is found, return “C# document found.” otherwise return “Sorry no C# document!”.
Sample Data:
("C# Exercises") -> "C# document found."
("Python Exercises") -> "Sorry no C# document!"
("Tutorial on c#") -> "C# document found."
Sample Solution:
C# Sharp Code:
using System;
using System.Text.RegularExpressions;
namespace exercises
{
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Define and initialize test strings
string text = "C# Exercises";
Console.WriteLine("Original string: " + text);
// Call the test method and display the result
Console.WriteLine(test(text));
// Update the test string
text = "Python Exercises";
Console.WriteLine("\nOriginal string: " + text);
// Call the test method and display the result
Console.WriteLine(test(text));
// Update the test string
text = "Tutorial on c#";
Console.WriteLine("\nOriginal string: " + text);
// Call the test method and display the result
Console.WriteLine(test(text));
}
// Method to check if the given text contains the term "C#" (case insensitive)
public static string test(string text)
{
// Check if the text contains the term "C#" (case insensitive)
bool flag = Regex.IsMatch(text, Regex.Escape("C#"), RegexOptions.IgnoreCase);
// Return different messages based on the presence of the term
if (flag)
{
return "C# document found.";
}
return "Sorry no C# document!";
}
}
}
Sample Output:
Original string: C# Exercises C# document found. Original string: Python Exercises Sorry no C# document! Original string: Tutorial on c# C# document found.
Flowchart:
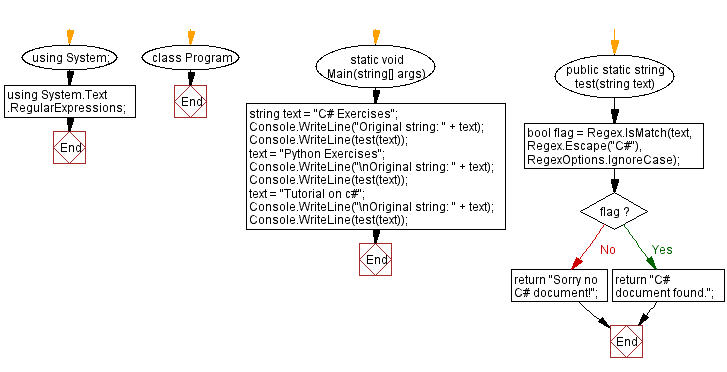
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous C# Sharp Exercise: Remove special characters from a given text.
Next C# Sharp Exercise: C# Sharp Regular Expression Exercises Home.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/csharp-exercises/re/csharp-re-exercise-9.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics