C#: Check for repeated occurrences of words in a string
Write a C# Sharp program to check for repeated occurrences of words in a given string.
Sample Data:
("C# C# syntax is highly expressive, yet it is is also simple and easy to to learn learn.") -> 3 matches found
("Red Green Green Black Black Green.") -> 2 matches found
Sample Solution:
C# Sharp Code:
using System;
using System.Text.RegularExpressions;
public class Test
{
public static void Main()
{
// Define a regular expression for repeated words.
Regex rx = new Regex(@"\b(?<word>\w+)\s+(\k<word>)\b",
RegexOptions.Compiled | RegexOptions.IgnoreCase);
// Define a test string.
string text = "C# C# syntax is highly expressive, yet it is is also simple and easy to to learn learn.";
//string text = "Red Green Green Black Black Green.";
// Display the original string.
Console.WriteLine("Original string: \n" + text);
// Find matches.
MatchCollection matches = rx.Matches(text);
// Report the number of matches found.
Console.WriteLine("{0} matches found in the said string:\n ", matches.Count);
// Report on each match.
foreach (Match match in matches)
{
GroupCollection groups = match.Groups;
Console.WriteLine("'{0}' repeated at positions {1} and {2}",
groups["word"].Value,
groups[0].Index,
groups[1].Index);
}
}
}
Sample Output:
Original string: C# C# syntax is highly expressive, yet it is is also simple and easy to to learn learn. 3 matches found in the said string: 'is' repeated at positions 42 and 45 'to' repeated at positions 69 and 72 'learn' repeated at positions 75 and 81
Flowchart:
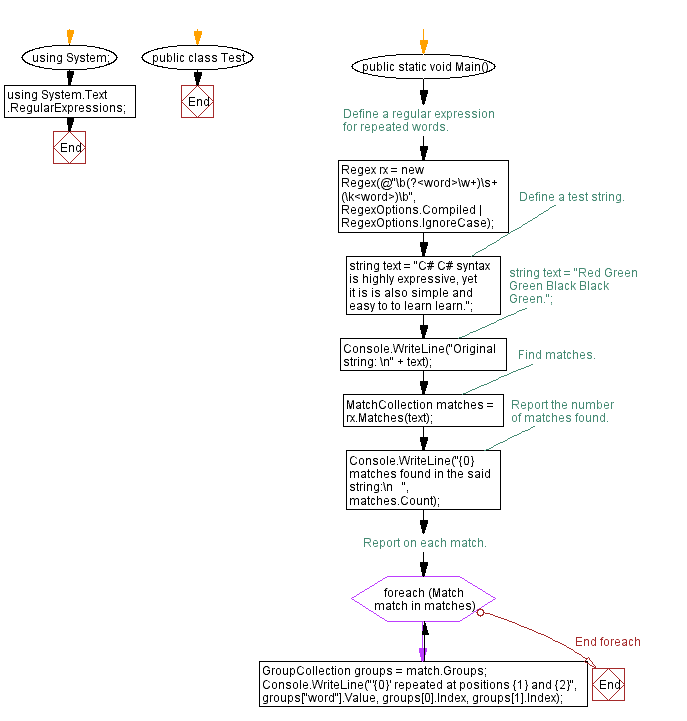
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous C# Sharp Exercise: Check two strings contain the same character pattern.
Next C# Sharp Exercise: Check whether a string represents a currency value.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics