C#: Check two strings contain the same character pattern
Write a C# Sharp program to check whether two given strings contain the same character pattern.
"AACC", "PPQQ" has the same pattern. "FFGG", "ADAD" does not have the same pattern.
Sample Data:
("AACC", "PPRR") -> True
("FFGG", "ADAD") -> False
Sample Solution:
C# Sharp Code:
using System;
using System.Text.RegularExpressions;
namespace exercises
{
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Initialize two string variables with some text content
string text1 = "AACC";
string text2 = "PPRR";
// Display the original strings
Console.WriteLine("Original strings: " + text1 + ", " + text2);
// Check if the lengths of the strings are the same before checking the character pattern
if (text1.Length == text2.Length)
{
// Check if the two strings contain the same character pattern
Console.WriteLine("Check if said two strings contain the same character pattern: " + test(text1, text2));
}
// Update the string variables with different content
text1 = "FFGG";
text2 = "ADAD";
// Display the updated strings
Console.WriteLine("\nOriginal strings: " + text1 + ", " + text2);
// Check if the lengths of the updated strings are the same before checking the character pattern
if (text1.Length == text2.Length)
{
// Check if the two updated strings contain the same character pattern
Console.WriteLine("Check if said two strings contain the same character pattern: " + test(text1, text2));
}
}
// Method to check if two strings contain the same character pattern
public static bool test(string text1, string text2)
{
// Loop through each character in the first string
for (int i = 0; i < text1.Length; i++)
{
// Check if the position of the character in both strings is the same
// If not, return false indicating different character patterns
if (text1.IndexOf(text1.Substring(i, 1), i + 1) != text2.IndexOf(text2.Substring(i, 1), i + 1))
return false;
}
// If all characters' positions match in both strings, return true indicating the same character pattern
return true;
}
}
}
Sample Output:
Original strings: AACC, PPRR Check said two strings contain the same character pattern: True Original strings: FFGG, ADAD Check said two strings contain the same character pattern: False
Flowchart:
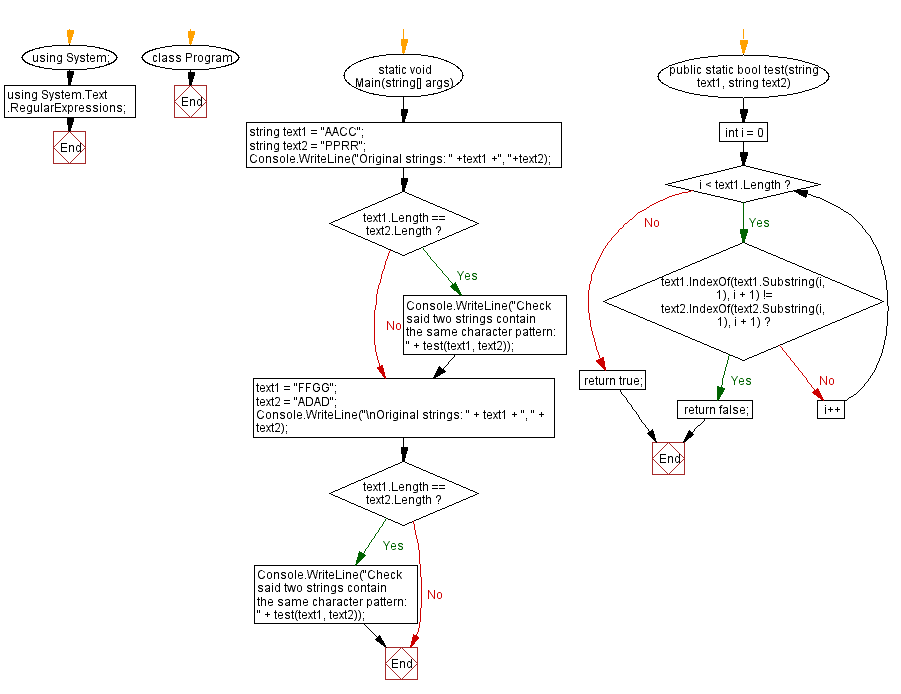
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous C# Sharp Exercise: Validate a password.
Next C# Sharp Exercise: C# Sharp Regular Expression Exercises Home.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.